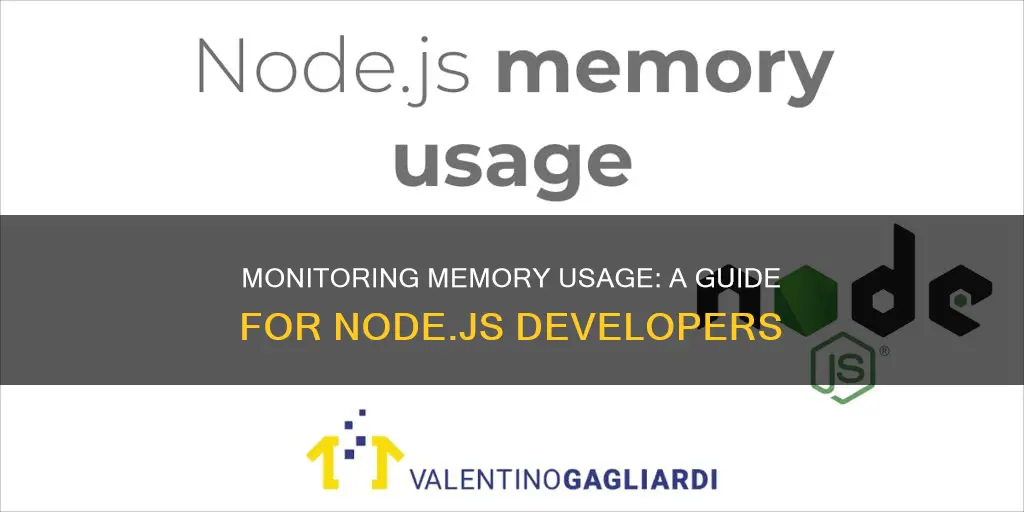
Memory leaks are a common issue in Node.js applications, and monitoring memory usage is essential to prevent crashes and optimise performance. Node.js provides built-in methods to gain insights into memory consumption, such as the process.memoryUsage() API, which offers details on Resident Set Size, heapTotal, heapUsed, external, and arrayBuffers. Additionally, tools like Clinic.js and Chrome DevTools can help visualise and analyse memory allocation over time, allowing developers to identify and fix memory leaks.
Characteristics | Values |
---|---|
Method to monitor memory usage | process.memoryUsage() |
Memory Heap division | Old space and new space |
Old space | Where older objects are stored |
New space | Where most objects are allocated |
Resident Set Size (rss) | Total memory allocated for the process execution |
heapTotal | Total size of the allocated heap |
heapUsed | Memory used during the execution of the process |
external | Memory used by C++ objects bound to JavaScript objects managed by V8 |
arrayBuffers | Memory allocated for all the Node.js Buffer instances |
What You'll Learn
Using the built-in process module
The built-in `process` module in Node.js provides the `memoryUsage()` method, which offers insights into the memory usage of the current Node.js process. This method returns an object with various memory-related metrics, including `rss` (Resident Set Size), `heapTotal` (total heap size), `heapUsed` (actual memory used during execution), and `external` (memory used by C++ objects bound to JavaScript objects).
Here's an example of how to use the `process` module to monitor memory usage:
Javascript
// Requiring the process module
Const process = require('process');
// Accessing memory usage information
Const memoryUsage = process.memoryUsage();
// Printing memory usage metrics
Console.log(memoryUsage);
Running this code will output an object with the memory usage details, such as:
{ rss: 23851008, heapTotal: 4907008, heapUsed: 2905912, external: 951886, arrayBuffers: 17574 }
The `process` module's `memoryUsage()` method is a powerful tool for monitoring memory usage in Node.js applications. It provides real-time insights into the memory consumption of the current process, making it easier to identify potential memory leaks or optimize memory usage.
Additionally, you can also use the `process.nextTick()` method to monitor maximum memory consumption. This method adds a callback to the next tick queue, allowing you to track memory usage over time and identify peak memory consumption.
Here's an example of how to use `process.nextTick()` to monitor maximum memory consumption:
Javascript
Let _maxMemoryConsumption = 0;
Let _dtOfMaxMemoryConsumption;
// Using process.nextTick() to monitor memory usage
Process.nextTick(() => {
Let memUsage = process.memoryUsage();
If (memUsage.rss > _maxMemoryConsumption) {
_maxMemoryConsumption = memUsage.rss;
_dtOfMaxMemoryConsumption = new Date();
}
});
// Logging maximum memory consumption on process exit
Process.on('exit', () => {
Console.log(
`Max memory consumption: ${_maxMemoryConsumption} at ${_dtOfMaxMemoryConsumption}`
;
});
In this example, the `_maxMemoryConsumption` variable keeps track of the maximum memory consumption, and `_dtOfMaxMemoryConsumption` stores the timestamp of when this maximum consumption occurred. The `process.nextTick()` callback compares the current memory usage (`memUsage.rss`) with the `_maxMemoryConsumption` value, and updates it if a new maximum is reached. Finally, when the process exits, the maximum memory consumption and its timestamp are logged to the console.
Monitoring Linux Memory Usage: A Comprehensive Guide
You may want to see also
Understanding memory allocation
Memory allocation is a critical aspect of software development, encompassing how memory is allocated and released within a program. In Node.js, memory management is facilitated by the V8 JavaScript engine, which includes a Resident Set of memory allocated to the program. This Resident Set comprises code, static data, stack, and heap memory.
The heap is a vital component of memory allocation, serving as the primary storage area for dynamic data and objects. It is divided into two main spaces: the old space and the new space. The old space accommodates older objects, typically those that have survived in the new space for a while. The new space, on the other hand, is where most objects are initially allocated. It is designed to be small and frequently cleaned to make room for new objects.
The V8 garbage collector plays a crucial role in memory management. It employs two algorithms: the Scavenger (Minor GC) and the Mark-Sweep & Mark-Compact (Major GC). The Scavenger algorithm focuses on short-lived objects in the new space, regularly checking for and removing unused objects, while the Mark-Sweep & Mark-Compact algorithms manage the old space, marking and sweeping away unused objects.
Monitoring Globe Broadband Usage: A Step-by-Step Guide
You may want to see also
Using the Node.js Memory Snapshot tool
The Node.js Memory Snapshot tool is a powerful way to monitor memory allocation and identify memory leaks in your Node.js application. Here's a step-by-step guide on how to use this tool effectively:
Instrument your application with Heapdump: Start by installing the Heapdump module, which provides a simple mechanism for producing V8 heap snapshots. You can install it using npm:
```
Npm install heapdump
```
Taking Heap Snapshots: There are several ways to take heap snapshots:
Using writeSnapshot method: Include the following line in your code to take a snapshot:
```javascript
Var heapdump = require('heapdump');
Heapdump.writeSnapshot();
```
Sending a SIGUSR2 signal (UNIX only): You can also send a SIGUSR2 signal to the process to trigger a snapshot. The snapshot will be saved with a timestamp in your current working directory. Ensure that your current working directory is writable by the process.
- Loading Snapshots into Chrome DevTools: Open Chrome DevTools and navigate to the "Profiles" tab. Right-click on the "Profiles" pane and select "Load" to load the snapshot file.
- Analysing Snapshots: Once you have loaded the snapshot, you can analyse it using the DevTools. The "Summary" view provides important data such as memory usage, shallow size, and retained size. The "Comparison" view is also useful for comparing multiple snapshots and identifying size differences.
- Strategies for taking snapshots: Memory leaks can occur slowly or on under-utilized code paths. It is recommended to take a baseline snapshot after your application has been running for a few minutes. You can then take subsequent snapshots as you notice memory usage increase. You can also take snapshots programmatically at specific intervals or based on memory usage thresholds.
- Analysing snapshot data: Compare two or more snapshots to identify memory leaks. Use the "Comparison" view in Chrome DevTools to see the size differences between snapshots. This will help you scope down the problem and identify the source of the memory leak.
- Understanding memory allocation: It is crucial to understand how V8 manages memory allocation. The memory heap is divided into two major spaces: "Old space" for older objects and "New space" for most newly allocated objects. The new space is further divided into "From space" and "To space". Understanding this structure will help you optimize memory usage and avoid leaks.
- Using Clinic Heap Profiler: The Clinic.js suite of tools includes the Heap Profiler, which helps uncover memory allocation by functions using Flamegraphs. You can install Clinic.js using npm:
```
Npm install -g clinic
```
By following these steps, you can effectively use the Node.js Memory Snapshot tool to monitor memory usage, identify memory leaks, and optimize your Node.js application's performance.
Monitoring Bandwidth Usage: Belkin Router Guide
You may want to see also
Using the Heap Profiler
The heap profiler acts on top of V8 to capture allocations over time. The Sampling Heap Profiler tracks the memory allocation pattern and reserved space over time. It is sampling-based, so its overhead is low enough to be used in production systems.
You can use the module heap-profiler to start and stop the heap profiler programmatically. Start the application with the following command:
$ node --inspect index.js
Then, connect to the dev-tools instance in Chrome and select the Memory tab, followed by the Allocation sampling option. Produce some load and stop the profiler. This will generate a summary of allocations based on their stack traces. You can then focus on the functions with more heap allocations.
The Heap Profiler - Allocation Timeline is similar to the Sampling Heap Profiler but will trace every allocation. It has higher overhead than the Sampling Heap Profiler, so it is not recommended for production.
N|Solid is a tool that includes sample heap profiling and heap object observability for main processes and worker threads. It can be used to trace memory leaks in your production applications on-demand. The powerful flame graph visualisation shows exactly where the problem lies by displaying the allocation stack trace and heap size.
Heap sampling avoids the overhead and high memory requirements of snapshots. It provides granular allocation details mapped to function names in your codebase, tracking incremental changes over time. This allows you to quickly identify the most memory-intensive areas of your application.
Monitoring Java Heap Usage: Tips for Performance Optimization
You may want to see also
Using the Sampling Heap Profiler
The Sampling Heap Profiler is a tool that can be used to monitor memory usage in Node.js applications. It is designed to be used programmatically and keeps track of the memory allocation pattern and reserved space over time. It has a low overhead, making it suitable for use in production systems.
Start the Node.js application with the following command:
> $ node --inspect index.js
- Connect to the dev-tools instance in Chrome.
- Select the "Memory" tab in the Chrome DevTools.
- Choose "Allocation sampling" from the options.
- Generate some load in your application to produce memory allocations.
- Once you have sufficient load, stop the profiler.
- The profiler will generate a summary of the memory allocations based on their stack traces.
By following these steps, you can identify functions with higher memory allocations and optimise them to improve the performance of your Node.js application.
Additionally, you can use the "heap-profile" module from npm to start, stop, and write snapshots of the Sampling Heap Profiler programmatically. This module adds support for the Sampling Heap Profiler in V8 and is designed to be lightweight for in-production use on servers.
CPU Performance Monitoring: Configuring for Optimum Usage
You may want to see also
Frequently asked questions
You can use the built-in process module's memoryUsage method to gain insight into the memory usage of the current Node.js process. This method offers details such as Resident Set Size (RSS), heapTotal, heapUsed, and external memory usage.
There are several tools available for monitoring memory allocation in Node.js applications. One option is to use the Chrome DevTools, which can provide a detailed summary of memory usage through a heap snapshot. Another tool is Clinic.js, which includes the Clinic Doctor tool to help diagnose performance issues related to memory consumption.
You can connect the Node.js debugger to a running process before it crashes to observe memory usage. This can be done by running the Node.js program in inspect mode, finding the process ID (PID), and then using the debugger to view the process's memory usage.