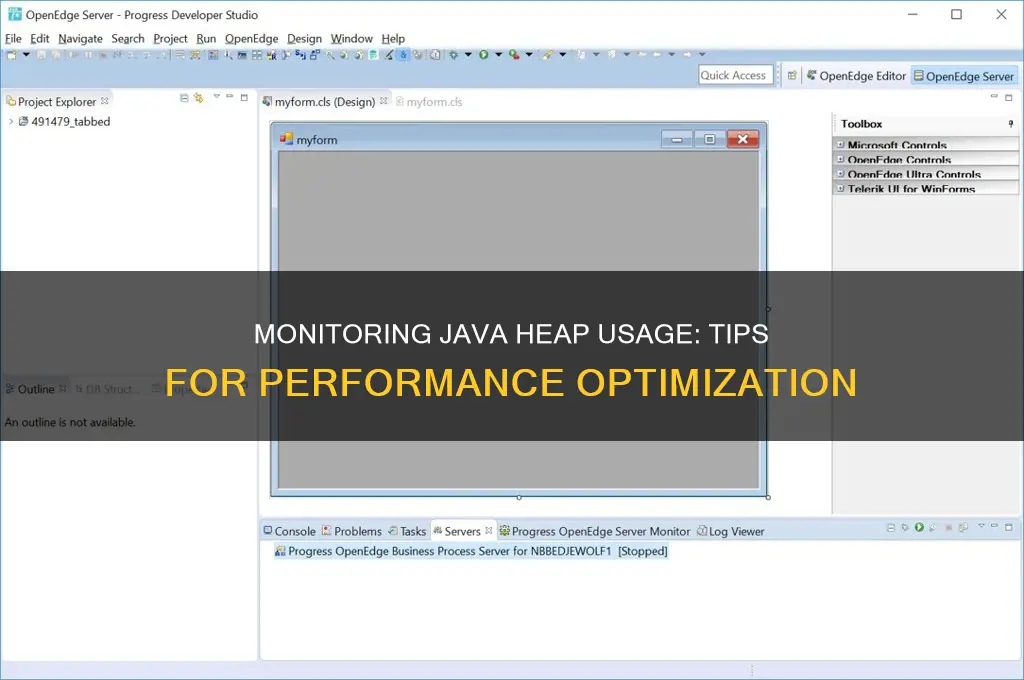
Monitoring Java heap usage is essential for optimising application performance and avoiding out-of-memory errors. The Java Virtual Machine (JVM) manages heap memory dynamically, but developers may need to intervene when memory issues arise. Various tools and techniques are available to monitor and troubleshoot Java heap usage, including manual methods and automated solutions. For example, the jcmd and jstat command-line utilities provide insights into heap and metaspace usage, while the -Xms and -Xmx flags allow explicit configuration of initial and maximum heap sizes. Developers can also leverage commercial and open-source tools like Datadog, Dynatrace, and VisualVM to monitor and analyse heap usage, identify memory leaks, and optimise application performance.
Characteristics | Values |
---|---|
Initial heap size | Configured by the -Xms flag |
Maximum heap size | Configured by the -Xmx flag |
Average heap usage | Can be monitored using Datadog's Java Agent |
Old generation size | Measured by the Usage.used metric |
Time spent in garbage collection | Can be tracked by querying the CollectionTime metric |
Garbage collection logs | Can be enabled using the -verbose:gc flag |
Heap space memory monitoring | builtin:tech.jvm.memory.pool.used and builtin:tech.jvm.memory.pool.commited |
Dynamically monitor Java heap size | totalMemory() |
Heap and metaspace info | jcmd command-line utility |
Current heap usage | Javacores txt files |
Heap occupancy | Heapdumps (Portable Heap Dump) files |
Mean or average heap usage | VerboseGC nativestderr.log output |
What You'll Learn
Use jcmd to find the heap and metaspace info of a running Java application
To find the heap and metaspace information of a running Java application, we can use the jcmd command-line utility.
First, find the process ID of a particular Java application using the jps command:
Bash
$ jps -l
73170 org.jetbrains.idea.maven.server.RemoteMavenServer36
4309 quarkus.jar
12070 sun.tools.jps.Jps
As shown above, the process ID for the Quarkus application is 4309. Now that we have the process ID, we can see the heap information:
Bash
$ jcmd 4309 GC.heap_info
4309: garbage-first heap total 206848K, used 43061K region size 1024K, 43 young (44032K), 3 survivors (3072K)
Metaspace used 12983K, capacity 13724K, committed 13824K, reserved 1060864K
Class space used 1599K, capacity 1740K, committed 1792K, reserved 1048576K
This app is using the G1 or garbage-first GC algorithm:
- The first line reports the current heap size as 202 MB (206848 K) – also, 42 MB (43061 K) is being used
- G1 regions are 1 MB, there are 43 regions marked as young, and 3 as survivors space
- The current capacity of the metaspace is around 13.5 MB (13724 K). From that 13.5 MB, around 12.5 MB (12983 K) is used. Also, we can have up to 1 GB of metaspace (1048576 K). Moreover, 13842 KB is guaranteed to be available for use by the Java virtual machine, also known as committed memory
- The last line shows how much of the metaspace is used to store class information
This output may change depending on the GC algorithm. For instance, if we run the same Quarkus app with ZGC via “-XX:+UnlockExperimentalVMOptions -XX:+UseZGC”:
Bash
ZHeap used 28M, capacity 200M, max capacity 1024M
Metaspace used 21031K, capacity 21241K, committed 21504K, reserved 22528K
As shown above, we’re using 28 MB of the heap and around 20 MB of metaspace. As of this writing, Intellij IDEA is still using the CMS GC with the following heap info:
Bash
Par new generation total 613440K, used 114299K
Eden space 545344K, 18% used
From space 68096K, 16% used
To space 68096K, 0% used
Concurrent mark-sweep generation total 1415616K, used 213479K
Metaspace used 423107K, capacity 439976K, committed 440416K, reserved 1429504K
Class space used 55889K, capacity 62488K, committed 62616K, reserved 1048576K
We can spot the classic generational nature of the CMS GC in the heap configuration.
Monitoring iPhone GB Usage: Tips and Tricks
You may want to see also
Use jstat to find out heap statistics
Jstat is a console tool that can be used to monitor the JVM heap and GC (Garbage Collection). It provides various statistics about the Java Virtual Machine (JVM), including information about heap memory usage, thread count, and classes.
To monitor heap usage using jstat, you can use the following command:
Jstat -gc
This command will provide detailed information about the current heap usage, including the size of the heap, the amount of used and free memory, and the number of garbage collection cycles.
For example, running `jstat -gc 12345 1000` will monitor the heap usage of the process with ID 12345, with a one-second interval between updates.
The output of the jstat command includes various metrics that can help you understand the heap usage:
- `S0C`, `S1C`, `EC`, and `OC` represent the current size of different memory regions within the heap.
- `S0U`, `S1U`, `EU`, and `OU` represent the used size of different memory regions within the heap.
- `MC` and `MU` represent the current and used size of the metaspace, which is used for storing class metadata.
By monitoring these values over time, you can track the heap usage and identify any potential memory leaks or performance issues.
Additionally, you can use the `-gccapacity` flag with jstat to get information about the maximum heap capacity:
Jstat -gccapacity
This command will provide the maximum capacity of each memory region within the heap, allowing you to understand the upper limits of memory allocation.
By combining the information from the `-gc` and `-gccapacity` options, you can gain a comprehensive understanding of your Java application's memory usage and make informed decisions about memory allocation and performance optimisation.
Monitoring Hydro Usage: Smart Metering and Water Efficiency
You may want to see also
Use jps to find out heap configuration options
The jps command can be used to find the process ID of a Java application. Once you have the process ID, you can use the jps command again with the -lv flag to find the heap configuration options for that application. This will display the static values specified during the application's runtime, such as the minimum and maximum heap sizes.
$ jps -l
4309 quarkus.jar -Xms200m -Xmx1g
In this example, the process ID for the Quarkus application is 4309. The heap configuration options are -Xms200m and -Xmx1g, indicating that the minimum heap size is set to 200 MB and the maximum heap size is set to 1 GB.
It's important to note that the jps command only provides static information about the heap configuration. If you want to know the current committed memory or other dynamic details, you will need to use additional tools or methods.
Monitoring Bandwidth Usage: Tips for Home Network Management
You may want to see also
Use ps from the procps package to find out heap configuration options
The `ps` command is a part of the `procps` package and can be used to find out heap configuration options. The command provides a snapshot of the current processes and their details such as process ID (PID), the terminal associated with the process (TTY), the cumulative CPU time, and the executable name.
The `ps` command accepts several options, including UNIX options, BSD options, and GNU long options. These options can be used to customize the output and select specific processes. For example, the -e option displays all processes on the system, while the -U option shows only the processes of a specific user.
To find heap configuration options, you can use the `ps` command with various options to list all processes and their memory usage details. For instance, using `ps -e` will display every process on the system along with their PIDs, TTYs, CPU times, and command names.
Additionally, the `ps` command can be combined with other tools to gain further insights. For instance, piping the output of `ps -e` to `less` allows for easier navigation through the extensive list of processes.
Bash
$ ps -e | less
PID TTY TIME CMD
1 ? 00:00:03 systemd
2 ? 00:00:00 kthreadd
3 ? 00:00:00 rcu_gp
4 ? 00:00:00 rcu_par_gp
6 ? 00:00:00 kworker/0:0H-events_highpri
Monitoring Linux Memory Usage: A Comprehensive Guide
You may want to see also
Use the /proc virtual filesystem to find out heap configuration options
The /proc virtual filesystem can be used to find out the heap configuration options of a Java application. First, the process ID of the Java application needs to be found. This can be done using the jps command. Once the process ID is known, the following command can be used to view the command-line entry for the application:
Bash
Cat /proc/[process_id]/cmdline
This will display the command-line options used to start the Java application, including any heap configuration options such as -Xms and -Xmx.
For example, if the process ID of a Quarkus application is 4309, the following command can be used to view the command-line entry:
Bash
Cat /proc/4309/cmdline
This will display the command-line options used to start the Quarkus application, including any heap configuration options.
Monitoring Data Usage: Tracfone's Easy Steps to Track
You may want to see also
Frequently asked questions
There are several tools available for monitoring Java heap usage, including Datadog, VisualVM, JConsole, jmap, jstat, Java-monitor, and more.
You can use the jcmd command-line utility to find the heap and metaspace-related information of a running Java application. First, use the jps command to find the process ID of your Java application. Then, use the jcmd command with the GC.heap_info option to see the heap information for that process ID.
There are several methods for monitoring Java heap usage in WebSphere, including generating Javacore dumps, enabling VerboseGC, using built-in PMI request metrics, and using tools such as IBM Support Assistant or Team Server.