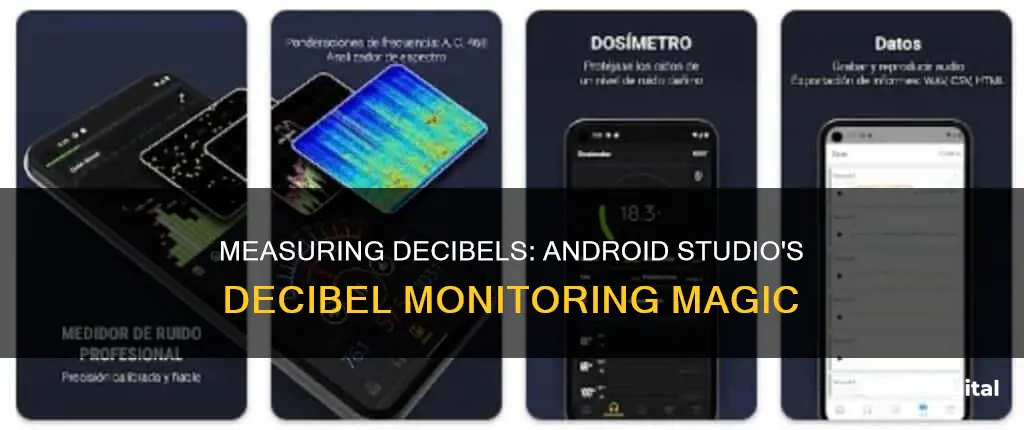
There are several ways to monitor decibels via Android Studio. For example, you can use an app such as Decibel X, which is available on both the App Store and Google Play, or Sound Meter, which is also available on both app stores. Alternatively, if you have an iPhone or Apple Watch, you can use the built-in Noise app to monitor your environment's noise levels. You can also monitor your headphone decibel levels through your iPhone by going to Settings, opening Control Center, and adding the Hearing option. If you're interested in developing your own app to monitor decibels, you can find sample code online, such as on Stack Overflow and GitHub. However, it's important to note that these devices and apps may not be as accurate as handheld sound level meters used by professionals.
Characteristics | Values |
---|---|
Purpose | To measure sound level in decibels |
Use cases | Detecting surrounding noise level, measuring headphone levels, monitoring noise in the environment |
Platforms | Android, iOS |
Tools | MediaRecorder, SoundMeter, Health app, Noise app, third-party apps |
Code languages | Java, HTML5/Javascript |
Accuracy | May be less accurate than handheld sound level meters used by professionals |
What You'll Learn
Decibel monitoring via Android Studio: the code
To monitor decibels via Android Studio, you can create a new Android application project and utilise specific code to measure the sound level in decibels. Here is a step-by-step guide with code examples:
Step 1: Create a New Project
Create a new Android application project in Android Studio and set up the necessary project configurations, such as project name, package name, and target SDK version.
Step 2: Add Necessary Permissions
In your project's `AndroidManifest.xml` file, add the following permission to access the device's microphone:
Xml
Step 3: Implement the Sound Meter Functionality
Create a new Java class, let's name it `SoundMeter.java`. In this class, you will implement the logic to measure the sound level in decibels. Here is an example code snippet:
Java
Package com.example.yourpackage;
Import android.app.Application;
Import android.media.MediaRecorder;
Import java.io.IOException;
Public class SoundMeter extends Application {
Static final private double EMA_FILTER = 0.6;
Private MediaRecorder mRecorder = null;
Private double mEMA = 0.0;
Public void start() {
If (mRecorder == null) {
MRecorder = new MediaRecorder();
MRecorder.setAudioSource(MediaRecorder.AudioSource.MIC);
MRecorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);
MRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB);
MRecorder.setOutputFile("/dev/null/");
Try {
MRecorder.prepare();
} catch (IllegalStateException | IOException e) {
E.printStackTrace();
}
MRecorder.start();
MEMA = 0.0;
}
}
Public void stop() {
If (mRecorder != null) {
MRecorder.stop();
MRecorder.release();
MRecorder = null;
}
}
Public double getAmplitude() {
If (mRecorder != null) {
Return (mRecorder.getMaxAmplitude() / 2700.0);
} else {
Return 0;
}
}
Public double getAmplitudeEMA() {
Double amp = getAmplitude();
MEMA = EMA_FILTER * amp + (1.0 - EMA_FILTER) * mEMA;
Return mEMA;
}
Step 4: Implement a User Interface
Design a user interface for your application using XML layout files. You can create a new Activity or Fragment to display the sound meter and provide a button for the user to start the sound measurement.
Step 5: Integrate the Sound Meter
In your Activity or Fragment class, where you have the user interface, import the `SoundMeter` class and create an instance of it. You can then call the `start()` method to begin measuring the sound level. Here is an example:
Java
Import com.example.yourpackage.SoundMeter;
Public class MyActivity extends AppCompatActivity {
Private SoundMeter mSoundMeter;
Private Button startButton;
@Override
Protected void onCreate(Bundle savedInstanceState) {
Super.onCreate(savedInstanceState);
SetContentView(R.layout.my_activity);
MSoundMeter = new SoundMeter();
StartButton = findViewById(R.id.start_button);
StartButton.setOnClickListener(new View.OnClickListener() {
@Override
Public void onClick(View v) {
MSoundMeter.start();
// You can update the UI here to display the measured decibel value
}
});
}
}
Step 6: Release Resources
Don't forget to release the resources when you are done with the sound measurement. Call the `stop()` method of the `SoundMeter` class to release the `MediaRecorder` object.
By following these steps and utilising the provided code examples, you can create an Android application that monitors decibels using Android Studio. Remember to adjust the code according to your specific project requirements and perform thorough testing to ensure the sound measurement is accurate and reliable.
Identifying High-DPI Monitors: What You Need to Know
You may want to see also
Decibel monitoring via Android Studio: the hardware
Decibel monitoring via Android Studio requires specific hardware components and configurations to function effectively. Here is an overview of the hardware aspects to consider:
Microphone:
The microphone is a crucial component for decibel monitoring as it captures the sound input. Android devices typically have built-in microphones that can be utilised for this purpose. However, it's important to note that the quality and sensitivity of the microphone can impact the accuracy of decibel measurements.
Audio Source:
The audio source setting determines the input source for the decibel monitoring application. In the code examples provided, the audio source is set to "MediaRecorder.AudioSource.MIC", indicating that the microphone will be used for capturing audio. This setting ensures that the application listens to the surrounding environment or the input from the user.
Audio Encoding and Output Format:
The audio encoding and output format settings configure how the captured audio data is encoded and formatted for processing. In the given code, the output format is set to "MediaRecorder.OutputFormat.THREE_GPP", which is a standard format for audio and video compression. The audio encoder is set to "MediaRecorder.AudioEncoder.AMR_NB", which is a narrowband speech coding standard. These settings ensure that the captured audio is appropriately encoded and formatted for further analysis.
External Hardware:
While most decibel monitoring applications rely solely on the device's built-in hardware, there may be cases where external hardware is utilised. This could include external microphones or audio input devices that provide additional functionality or improved accuracy. However, integrating external hardware may require additional code and configuration to ensure compatibility and proper data processing.
Device Compatibility:
It's important to consider the compatibility of the decibel monitoring application with different Android devices. Variations in hardware components, such as microphones and processors, can impact the accuracy and performance of the application. Therefore, testing and optimising the application for a range of devices is crucial to ensure consistent and reliable results.
In summary, decibel monitoring via Android Studio relies on a combination of built-in hardware components, such as microphones, and proper configuration of audio settings. External hardware can also be incorporated, but it requires additional considerations for compatibility and data processing. Ensuring device compatibility and testing across a range of devices will help provide accurate and reliable decibel monitoring functionality.
Running Performance Monitor Remotely: A Step-by-Step Guide
You may want to see also
Decibel monitoring via Android Studio: accuracy
Decibel monitoring via Android Studio is possible, and there are several apps available that can help you do this. However, it's important to note that these apps may not provide the same level of accuracy as handheld sound level meters used by professionals.
Built-in Features on iOS Devices
IOS devices like iPhones and Apple Watches have built-in features for tracking decibels in certain situations. You can monitor your headphone levels by going to Settings, opening Control Center, and adding the Hearing option. Once connected, tap the Hearing icon to see the real-time decibel level of your headphones. The Health app on your iPhone can also help you monitor your headphone decibel levels over time.
The Apple Watch is equipped with the Noise app, which continuously monitors ambient noise levels and sends alerts if it detects potentially harmful sound levels. The data is synced with the Health app to help you track your exposure to loud noises over time.
Sound Meter Apps
There are several sound meter apps available for both Android and iOS devices that can provide more features and functionality for decibel monitoring. Here are some popular options:
- Decibel X: This app turns your smartphone into a pre-calibrated, accurate, and portable sound level meter with a standard measurement range from 30 to 130 dB.
- Decibel Pro: An app that allows you to measure noise and calibrate audio equipment. It includes a noise dosimeter, spectrum analyzer, hearing test, and more.
- Sound Meter (Noise Detector): This app alerts you when dangerous noise levels are present, helps you test your hearing, and provides music for relaxation and focus. It can be used to test noise in various environments to ensure they meet industry standards.
- Too Noisy: Specifically designed for measuring noise levels in classrooms, this app helps teachers control the noise level with a simple and engaging interface.
- NIOSH Sound Level Meter: Developed by the National Institute for Occupational Safety and Health, this app is ideal for anyone working in noisy environments. It raises awareness about noise levels, helps make informed decisions about hearing health, and determines when hearing protection is necessary.
Developing Your Own App
If you're interested in developing your own decibel monitoring app, there are resources available, such as code snippets and guides, that can help you get started. However, it's important to note that calculating decibel values accurately can be complex, and you may need to consider factors such as peak versus RMS power levels and automatic gain control.
Finding Files Outside Your Monitor: A Comprehensive Guide
You may want to see also
Decibel monitoring via Android Studio: calibration
Decibel monitoring via Android Studio requires calibration for accurate results. Here is a guide on how to achieve this:
Firstly, it is important to understand the limitations of Android devices in measuring decibels. While it is possible to measure external sound using the device's microphone, measuring sound output from earphones/headphones is more complex due to varying inherent values such as impedance, resistance, and material.
To calibrate your Android device for decibel monitoring, you can follow these steps:
- Install a reliable sound meter app: Choose an app that is known for its accuracy and has pre-calibrated measurements, such as "Decibel X" or "Sound Meter".
- Custom calibration: While these apps provide pre-calibrated measurements, custom calibration is recommended for higher precision.
- Use an external reference: You will need a real external device or a calibrated sound meter as a reference point. Adjust the trimming calibration on your app until the reading matches the reference.
- Test in different environments: Calibrate your device in different locations with varying noise levels to ensure accuracy across a range of sound pressures.
- Compare with professional devices: If possible, compare the readings from your Android device with those from a professional sound level meter used by experts. This will help validate the accuracy of your calibration.
- Account for device variations: Different Android devices may have slight variations in microphone sensitivity and audio processing. Compare results with other similar devices to ensure consistency.
- Regularly re-calibrate: Over time, the microphone and audio components of your device may experience wear and tear, affecting accuracy. Re-calibrate periodically to maintain reliable results.
By following these steps, you can effectively calibrate your Android device for decibel monitoring, ensuring more accurate measurements of sound levels in your environment.
It is important to note that even with careful calibration, smartphone decibel meter apps may not match the accuracy of professional handheld sound level meters used by experts.
Understanding Monitor Quality: Finding Your SRGB
You may want to see also
Decibel monitoring via Android Studio: third-party apps
There are several third-party apps available for monitoring decibels via Android Studio. Here is a list of some popular options:
Apps for Both Android and iOS
- Decibel X: dB Meter: This app turns your smartphone into a pre-calibrated, accurate, and portable sound level meter. It has a standard measurement range from 30 to 130 dB and features a nicely designed, intuitive user interface.
- Decibel Pro: dB Sound Level Meter: This app allows you to measure noise at home or in the workplace and calibrate home or professional audio equipment. It includes a noise dosimeter, spectrum analyzer with RTA, FFT, and Spectogram, as well as a hearing test.
- Sound Meter (Noise Detector): Use this app to be alerted when dangerous noise levels are present, test your hearing, and listen to music for relaxation and focus. It can also be used to test noise in buildings and cars to ensure they meet industry standards.
- Too Noisy Pro: This app is designed specifically for measuring noise levels in environments with groups of children. Teachers can use it to monitor and control the noise level in classrooms. The interface is simple and designed to be engaging for children, with a fun and graphical display of noise levels.
Apps for Android Only
- Sound Level Meter: This app uses your smartphone's microphone to detect sound and display it in decibels. It also displays the last 30 seconds of data on an easy-to-read graph. However, sounds above 100 dB are not recognized due to the limitations of Android device microphones.
- Sound Meter and Noise Detector: Like most sound meter apps, this app uses your smartphone microphone to measure the sound in your environment. The decibel level is displayed on an easy-to-read dial, and the history function indicates how long you've been exposed to the noise, along with the noise reference (e.g., noisy street or quiet library).
It's important to note that these apps may not be as accurate as handheld sound level meters used by professionals, and they do not meet ANSI and OSHA requirements for certain specific use cases.
Calibrating Your ASUS 144Hz Monitor: A Step-by-Step Guide
You may want to see also
Frequently asked questions
Yes, there are several apps available on the Google Play Store that can measure sound levels in decibels. Examples include "Sound Meter", "Sound Meter and Noise Detector", and "Decibel X".
Yes, if you have an Android phone, you can use the "Sound Meter" app to measure the sound levels in your environment. Additionally, if you have an iPhone or Apple Watch, you can use the built-in "Hearing" and "Noise" apps to track decibel levels.
While these apps can be useful, they may not be as accurate as handheld sound level meters used by professionals. They also do not meet ANSI and OSHA requirements for evaluating workplace noise or conducting hearing tests.
Yes, you can create such an app using Java code. There are online resources and code examples available that can help you get started with developing this type of app.
It is unclear if this is possible. While some sources suggest that native Android/Java code is required, others provide code snippets in Java that may be adaptable for HTML5/JavaScript.