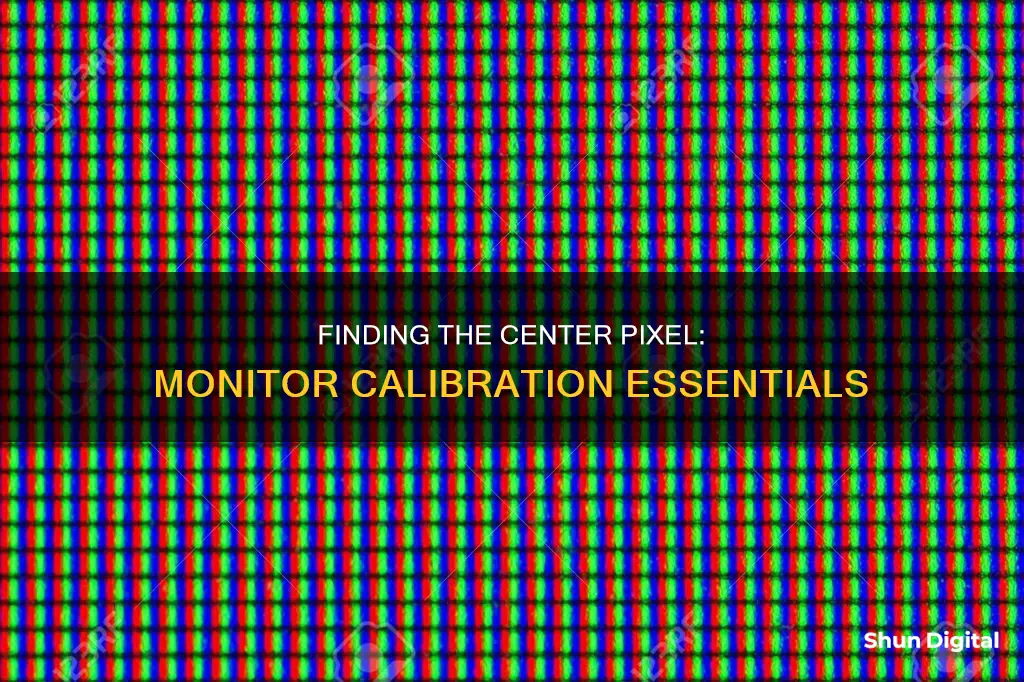
Finding the centre pixel of a monitor can be done in a variety of ways, depending on the hardware and software in use. For example, on a Windows system, one can detect full-screen mode and prompt the user to press F11 to maximise the window. On a Mac, there is no true full-screen mode, but the height of the stuff at the top can be calculated and subtracted to find the true centre relative to the monitor. In Java, one can use the getWidth() and getHeight() methods to find the centre.
Characteristics | Values |
---|---|
Method | Divide the screen width and height by 2 |
Use built-in variables for screen width and height | |
Use Screen.WorkingArea |
|
Set the StartPosition property of the main WinForm to CenterScreen |
|
Use System.Windows.Forms.Screen.PrimaryScreen.Bounds |
|
Use System.Windows.Forms.Screen.PrimaryScreen.WorkingArea |
|
Use Window#setLocationRelativeTo(null) |
|
Use Window#setLocationRelativeTo(null) and Timr's solution |
|
Use getWidth()/2 and getHeight()/2 |
|
Use A_ScreenWidth // 2 and A_ScreenHeight // 2 |
|
Use WinGetPos |
|
Use SysGet |
What You'll Learn
Finding the centre pixel on a Mac
Using the Screenshot Function:
- Press ⇧⌘4 to activate the screenshot function.
- This will show the coordinates of your cursor on the screen.
- Move your cursor to the desired location, and note down the coordinates.
- To find the centre pixel, you will need to calculate the middle point between the top-left and bottom-right coordinates of your screen.
Using an Image in Preview:
- Open the image in Preview.
- Click on the "Show Inspector" option in the Tools menu.
- Select the tab with a ruler icon. This will display the image coordinates.
- Ensure that your image is zoomed to 100% to get accurate measurements.
- Drag from the desired pixel to the upper-left corner of the screen.
- This will create a selection rectangle, and the size of this rectangle will be the same as the pixel coordinates you're trying to find.
Using DigitalColor Meter:
- Open the DigitalColor Meter application.
- Enable the "Show Mouse Location" option in the View menu.
- This will display the coordinates of your cursor on the screen.
- Move your cursor to the centre of the screen, and note down the coordinates.
Using JavaScript:
If you are comfortable with coding, you can use JavaScript to find the centre pixel programmatically. Here is an example code snippet:
Javascript
Let offset = window.screen.height - window.innerHeight;
This code calculates the offset from the top of the screen to the top of the viewport. By subtracting this offset from the screen height, you can find the y-coordinate of the centre pixel. A similar calculation can be done for the x-coordinate.
Monitoring Data Usage: WWDRT for Precise Device Tracking
You may want to see also
Finding the centre pixel on Windows
There are a few ways to find the centre pixel of a monitor on a Windows device.
Using Point Position
Point Position is a freeware tool that allows you to pick the coordinates of any point on your screen. To use it, simply point one of the four corner arrows at the desired spot on your screen and click the corresponding button. The text entries will then display the exact coordinates.
Using Powershell
You can also use a Powershell script with System.Windows.Forms.Cursor to find the coordinates of your cursor. The script is as follows:
Add-Type -AssemblyName System.Windows.Forms
While (1) {
$X = [System.Windows.Forms.Cursor]::Position.X
$Y = [System.Windows.Forms.Cursor]::Position.Y
Write-Host -NoNewline "X:$X | Y: $Y" }
Using Cursor Position
Cursor Position is a software that allows you to find the exact X and Y position of your cursor, even as you move it. It also tells you how many pixels you have travelled across the screen.
Centering a JFrame
If you are using a JFrame, you can centre it on the screen by calling `Window#setLocationRelativeTo(null)`. Alternatively, you can use the following method to centre a JFrame on the screen:
Public static void moveToCenterScreen(JFrame frame) {
Toolkit kit = frame.getToolkit();
GraphicsDevice[] gs = GraphicsEnvironment.getLocalGraphicsEnvironment().getScreenDevices();
Insets in = kit.getScreenInsets(gs [0].getDefaultConfiguration());
Dimension d = kit.getScreenSize();
Int max_width = (d.width - in.left - in.right);
Int max_height = (d.height - in.top - in.bottom);
Frame.setLocation((int) (max_width - frame.getWidth()) / 2, (int) (max_height - frame.getHeight() ) / 2);
Cleaning Your LCD Monitor: Windex Spray Guide
You may want to see also
Using AutoHotkey to find the centre pixel
AutoHotkey is a free, open-source scripting language for Windows that can be used to automate repetitive tasks and create custom keyboard shortcuts. In this case, we are interested in using AutoHotkey to find the centre pixel of a monitor.
Single Monitor Setup
To find the centre pixel of a single monitor, you can use the following code:
Xx := A_ScreenWidth // 2
Yy := A_ScreenHeight // 2
Here, `A_ScreenWidth` and `A_ScreenHeight` are built-in variables in AutoHotkey that store the width and height of the screen, respectively. By dividing these values by 2, we can find the x and y coordinates of the centre pixel.
Multi-Monitor Setup
If you have a multi-monitor setup, you can use the `SysGet` command to get the coordinates of each monitor. The following code demonstrates how to do this:
Loop, %MonAct% {
SysGet, coord%A_Index%, MonitorWorkArea, %A_Index%
}
In this code, `%MonAct%` represents the number of active monitors. The `Loop` statement iterates through each monitor, and the `SysGet` command retrieves the coordinates of each monitor's work area. The coordinates are stored in an array, `coord%A_Index%`, where `%A_Index%` is the index of the current monitor.
Finding the Centre of the Active Window
If you want to find the centre of the monitor with the active window, you can use the following code:
WinGetPos, ActX, ActY, ActW, ActH, A
Loop, %MonAct% {
SysGet, Mon%A_Index%, Monitor, %A_Index%
}
Loop, %MonAct% {
If (compare(ActX, ActY, ActW, ActH, Mon%A_Index%Left, Mon%A_Index%Top, Mon%A_Index%Right, Mon%A_Index%Bottom)) {
Break
}
}
Here, the `WinGetPos` command retrieves the coordinates of the active window, and the `SysGet` command retrieves the coordinates of each monitor. The `compare` function checks if the active window's coordinates are within the bounds of each monitor, and if a match is found, the loop breaks and the centre coordinates are calculated as follows:
I := a + c // 2 ; get active window center H
J := b + d // 2 ; get active window center V
Finding the Centre Pixel of a Specific Area
If you want to find the centre of a specific area on the screen, you can use the `graphicsearch` function provided by the AutoHotkey community. Here is an example code snippet:
OGraphicSearch := new graphicsearch()
Area := {x:135, y:294, x2:778, y2:845}
NearbyDistance := 90
ResultObj := oGraphicSearch.search(cubeGraphic, {err0: .16, x1:135, y1:294, x2:778, y2:845})
In this code, `oGraphicSearch` is an instance of the `graphicsearch` class, and `area` defines the bounds of the specific area on the screen. The `nearbyDistance` variable specifies the distance threshold for considering pixels to be "nearby". The `resultObj` variable stores the result of the search, which can then be used to calculate the centre of the area.
Is Your Holter Monitor On? How to Tell
You may want to see also
Finding the centre pixel in Java
Finding the centre pixel of a monitor can be done by first determining the screen's resolution, which is typically expressed in pixels. The centre pixel can then be calculated by dividing the width and height of the screen by two to get the x and y coordinates, respectively.
In Java, you can use the following code to find the centre pixel of an image:
Java
Import java.awt.Color;
Import java.awt.image.BufferedImage;
Import java.io.File;
Import java.io.IOException;
Import javax.imageio.ImageIO;
Public class PixelFinder {
Public static void main(String[] args) {
// Replace "image.bmp" with your image file
File imageFile = new File("image.bmp");
Try {
BufferedImage image = ImageIO.read(imageFile);
Int width = image.getWidth();
Int height = image.getHeight();
Int centreX = width / 2;
Int centreY = height / 2;
System.out.println("Centre pixel coordinates: (" + centreX + ", " + centreY + ")");
} catch (IOException e) {
E.printStackTrace();
}
}
}
This code reads an image file, gets its width and height, and then calculates the x and y coordinates of the centre pixel by dividing the width and height by two, respectively. Finally, it prints out the coordinates of the centre pixel.
Note that this code assumes the image is in a standard format like BMP, JPEG, or PNG, and that you have the necessary permissions to read the image file.
Additionally, if you are working with a monochrome bitmap that contains a single, randomly rotated rectangle, you can use more complex algorithms to find the corner pixels and, subsequently, the centre pixel. One approach involves finding the bounding box of the rectangle and then adjusting its corners clockwise until they are black. Another method involves scanning the image from the top, row by row, to find a black run and then repeating this process from the bottom, left, and right to identify eight corner candidates.
Fixing LCD Monitors: DIY Guide to Screen Repair
You may want to see also
Finding the centre pixel in C#
To find the centre pixel of a monitor in C#, you can use the following approaches:
Approach 1:
You can calculate the centre pixel coordinates by finding the screen resolution. For example, if your screen resolution is 640x480 pixels, the centre coordinates would be (320, 240). You can use the System.Windows.Forms.Screen.PrimaryScreen.Bounds property to get the screen bounds and then calculate the centre coordinates based on the width and height.
Approach 2:
Another way is to use the StartPosition property of your main WinForm and set it to CenterScreen. This will automatically centre your program on the screen. If you need to adjust the position further, you can modify the Top and Left properties to move the form relative to the screen centre.
Approach 3:
If you want to find the centre of an object within an image, you can calculate the centre of gravity of the pixels. This involves summing the x and y coordinates of the pixels and then dividing by the total number of pixels. This will give you the centre coordinate of the object.
Approach 4:
In some cases, you may need to find the new coordinate of a pixel after rotating an image. To do this, you need to take into account any translation transformations applied to the image. You can use trigonometric functions, such as cosine and sine, to calculate the new coordinates based on the angle of rotation and the original pixel position.
Here's an example code snippet that demonstrates how to find the centre of the screen in C#:
Csharp
Var screensize = System.Windows.Forms.Screen.PrimaryScreen.Bounds;
Var programsize = Bounds;
Location = new Point((screensize.X - programsize.X) / 2, (screensize.Y - programsize.Y) / 2 - 10);
Monitoring Data Usage on Your iPad: A Guide
You may want to see also
Frequently asked questions
You can detect full-screen mode and prompt the user to press F11 to make everything easier to find.
On a Mac, there is no such thing as full-screen mode, but you can maximise your screen so that the address bar and buttons are stuck to the top.
You can set the StartPosition property of your main WinForm to CenterScreen and then play with the Top and Left properties to add or subtract the required number of pixels.
You can use the following code:
```
public static void moveToCenterScreen(JFrame frame) {
Toolkit kit = frame.getToolkit();
GraphicsDevice[] gs = GraphicsEnvironment.getLocalGraphicsEnvironment().getScreenDevices();
Insets in = kit.getScreenInsets(gs [0].getDefaultConfiguration());
Dimension d = kit.getScreenSize();
int max_width = (d.width - in.left - in.right);
int max_height = (d.height - in.top - in.bottom);
frame.setLocation((int) (max_width - frame.getWidth()) / 2, (int) (max_height - frame.getHeight()) / 2);
```