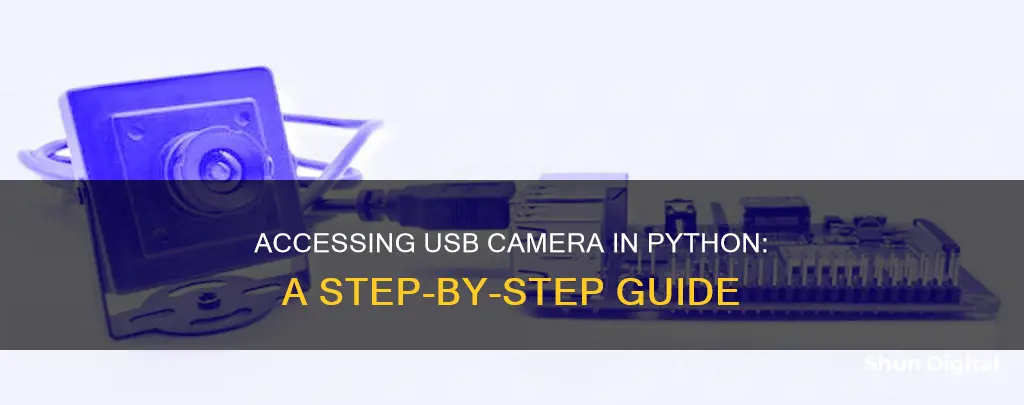
There are several ways to access a USB camera in Python. One common method is to use the OpenCV library, which is an open-source image processing bundle that supports a wide range of image processing functions. By installing OpenCV and running a few lines of code, you can access and stream video from a USB camera. Additionally, other methods such as using the fswebcam package or the ffmpeg tool can be used to control and capture images from a USB webcam.
What You'll Learn
Using OpenCV with Python
OpenCV, short for Open Source Computer Vision Library, is an open-source computer vision and machine learning software library. It supports a wide variety of programming languages, including Python, and can process images and videos to identify objects, faces, and even handwriting.
OpenCV-Python is the Python API for OpenCV, combining the qualities of the OpenCV C++ API and the Python language. It is a library of Python bindings designed to solve computer vision problems.
To use OpenCV with Python, you will need to install and set up the library. Here is a general guide on how to do so:
First, ensure you have the necessary dependencies installed. For Ubuntu, you can use the following commands:
Libav video input/output development libraries
$ sudo apt-get install libavformat-dev libavutil-dev libswscale-dev
Video4Linux camera development libraries
$ sudo apt-get install libv4l-dev
OpenGL development libraries (for graphical windows)
$ sudo apt-get install libglew-dev
GTK development libraries (for graphical windows)
$ sudo apt-get install libgtk2.0-dev
Install the OpenCV python library
$ sudo apt-get install python-opencv
Next, download the OpenCV source code and unzip it:
$ wget https://github.com/opencv/opencv/archive/4.6.zip
$ unzip opencv-4.6.zip
$ cd opencv-4.6
Create a build directory and navigate to it:
$ mkdir build && cd build
Configure the build using CMake:
$ cmake -D CMAKE_BUILD_TYPE=RELEASE -D WITH_TBB=OFF -D BUILD_TBB=OFF -D WITH_V4L=ON -D WITH_LIBV4L=OFF -D BUILD_TESTS=OFF -D BUILD_PERF_TESTS=OFF ..
Finally, compile and install OpenCV:
$ sudo make j4 install
This will install the OpenCV libraries on your system.
To use OpenCV with Python to access a USB camera, you can use the following code as a starting point:
Python
Import cv2
Open the USB camera device
Cap = cv2.VideoCapture(0)
Check if the camera is opened successfully
If not (cap.isOpened()):
Print("Could not open video device")
Set the resolution of the camera
Cap.set(cv2.cv.CV_CAP_PROP_FRAME_WIDTH, 640)
Cap.set(cv2.cv.CV_CAP_PROP_FRAME_HEIGHT, 480)
Capture frames from the camera and display them in a preview window
While(True):
# Capture frame-by-frame
Ret, frame = cap.read()
# Display the resulting frame
Cv2.imshow('preview', frame)
# Wait for a user input to quit the application
If cv2.waitKey(1) & 0xFF == ord('q'):
Break
Release the camera and close all windows
Cap.release()
Cv2.destroyAllWindows()
This code will open the USB camera, set the resolution, capture frames, display them in a preview window, and allow the user to quit the application by pressing the 'q' key.
Note that you may need to adjust the camera index (the number passed to `cv2.VideoCapture`) if your USB camera is not detected. The default index is typically 0 for built-in cameras, but for USB cameras, it may be 1 or 2. You can use the following script to find all available camera indices:
Python
Import cv2
Import numpy as np
All_camera_idx_available = []
For camera_idx in range(10):
Cap = cv2.VideoCapture(camera_idx)
If cap.isOpened():
Print(f'Camera index available: {camera_idx}')
All_camera_idx_available.append(camera_idx)
Cap.release()
Can My Camera Spy on Me?
You may want to see also
Finding the camera index
To find the camera index of a USB camera in Python, you can use the following script:
Python
Import cv2
Import numpy as np
All_camera_idx_available = []
For camera_idx in range(10):
Cap = cv2.VideoCapture(camera_idx)
If cap.isOpened():
Print(f'Camera index available: {camera_idx}')
All_camera_idx_available.append(camera_idx)
Cap.release()
This script will iterate through camera indices from 0 to 9 and attempt to open a camera stream for each index using the `cv2.VideoCapture` class. If a camera stream can be successfully opened for a particular index, that index will be added to the `all_camera_idx_available` list, and a message will be printed to the console indicating the available camera index. After checking all indices, the camera stream is released.
The result will be a list of all available camera indices, which could include indices other than 0, 1, or 2. For example, the list might look like ` [0,1,2,...]` if the default integrated camera and multiple USB cameras are connected and recognised.
Finding and Using Your Windows 7 Camera
You may want to see also
Installing OpenCV in Anaconda
To access a USB camera using Python, OpenCV (Open-Source BSD licensed image processing bundle) is a commonly used application.
Now, to install OpenCV in Anaconda, here is a step-by-step guide:
Step 1: Create a New Anaconda Environment
It is always a good practice to create a separate environment for each project to avoid conflicts between package versions. For an OpenCV project, open your terminal or Anaconda Prompt and run the following command:
`conda create --name opencv_env python=3.8`
This command creates a new environment named opencv_env with Python 3.8 as the default interpreter.
Step 2: Activate the Environment
To use the newly created environment, activate it by running the following command:
`conda activate opencv_env`
Your terminal prompt should now display the name of the activated environment.
Step 3: Install OpenCV
With Anaconda, installing OpenCV is straightforward. Run the following command:
`conda install -c conda-forge opencv`
This command installs OpenCV from the conda-forge channel, which provides a wide range of packages for scientific computing.
Step 4: Verify the Installation
To verify that OpenCV is installed correctly, write a simple Python script to display an image. Create a new Python file named `test_opencv.py` and add the following code:
Python
Import cv2
Read an image
Img = cv2.imread('image.jpg')
Display the image
Cv2.imshow('Image', img)
Cv2.waitKey(0)
Cv2.destroyAllWindows()
Make sure to replace 'image.jpg' with the path to an image file of your choice.
Now, run the script using the following command:
`python test_opencv.py`
If OpenCV is installed correctly, a window should appear displaying the image you specified.
Congratulations! You have successfully installed OpenCV in an Anaconda environment and can now explore computer vision and image processing using Python. Remember to activate the `opencv_env` environment for projects requiring OpenCV.
Trail Cameras: Can Deer See Them?
You may want to see also
Controlling a USB webcam with Python
To control a USB webcam with Python, you will need to use a suitable library, such as OpenCV, Pygame, or Fswebcam. Here is a step-by-step guide on how to do it:
Step 1: Install the Required Library
First, you need to install the library of your choice. For this example, we will use OpenCV, which can be installed using the following command:
Pip install opencv-python
Step 2: Import the Necessary Modules
In your Python script, import the required modules:
Python
Import cv2
Step 3: Set Up the Webcam Object
Create a `VideoCapture` object and pass the device ID of your webcam. If you have multiple cameras or your webcam is not detected as `0`, you may need to adjust this number.
Python
Cap = cv2.VideoCapture(0)
Step 4: Capture Frames and Process Them
Use a loop to continuously capture frames from the webcam and process them as needed. Here is a basic example of displaying the frames in a preview window:
Python
While True:
Ret, frame = cap.read()
Cv2.imshow('preview', frame)
If cv2.waitKey(1) & 0xFF == ord('q'):
Break
Step 5: Release the Webcam and Close Windows
After you are done capturing and processing frames, release the webcam and close all open windows:
Python
Cap.release()
Cv2.destroyAllWindows()
Step 6: Run Your Script
Save your script and run it using the Python interpreter. You should now be able to see the webcam feed in the preview window.
Advanced Usage
With OpenCV, you can also set various properties of your webcam, such as the resolution:
Python
Cap.set(cv2.cv.CV_CAP_PROP_FRAME_WIDTH, 640)
Cap.set(cv2.cv.CV_CAP_PROP_FRAME_HEIGHT, 480)
Additionally, you can use other libraries like Pygame for more advanced tasks, such as object tracking:
Python
Import pygame
Import pygame.camera
Pygame.init()
Pygame.camera.init()
Cam = pygame.camera.Camera("/dev/video0", (320, 240))
Cam.start()
Lane Watch Camera: When Did Honda Introduce This Feature?
You may want to see also
Setting up multiple USB webcams
When it comes to controlling the webcams with Python, you can use the OpenCV library. To record a single image from a specific camera, you can use code similar to the following:
Python
Import cv2
Cam = cv2.VideoCapture(device_id)
While True:
Ret, image = cam.read()
Cv2.imshow('Imagetest', image)
K = cv2.waitKey(1)
If k != -1:
Break
Cv2.imwrite('/path/to/image.jpg', image)
Cam.release()
Cv2.destroyAllWindows()
In this code, you would replace `device_id` with the ID of the camera you want to use (0 for the first camera, 1 for the second, and so on).
It's worth noting that USB 2.0 webcams have a limited bandwidth of 480 Mbit/s, so if you're using multiple cameras, the resolution, frame rate, and compression will be important factors to consider.
A Simple Guide to Viewing IPUX Cameras
You may want to see also
Frequently asked questions
Although it is recommended to use a Raspberry Pi camera module, you can still use a USB camera. First, plug in the camera and check if the Raspberry Pi recognises it by entering lsusb in the terminal. Then, to take an image with a certain resolution and hide the standard banner, enter the following code:
```
sudo apt install fswebcam
fswebcam -r 1280x720 --no-banner /images/image1.jpg
```
You can use the following code to access your USB camera:
```
import cv2
cap = cv2.VideoCapture(0)
if not (cap.isOpened()):
print("Could not open video device")
while(True):
ret, frame = cap.read()
cv2.imshow('frame',frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
You can use the following code to take a picture from a USB camera:
```
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
(grabbed, frame) = cap.read()
cv2.imshow('img1', showimg) # display the captured image
time.sleep(0.3) # Wait 300 miliseconds
image = 'C:\Users\Lukas\Desktop\REMOVE\capture.png'
cv2.imwrite(image, frame)
```
You can use the Anaconda environment to access your USB camera on Windows. First, install OpenCV in your Anaconda environment by running the following:
```
(base) C:\WINDOWS\system32>conda install -c conda-forge opencv
```
Then, open the Spyder editor and run the following code:
```
-*- coding: utf-8 -*-
"""
Created on Sun May 3 20:50:56 2020
@author: Ilias Sachpazidis
"""
import cv2
print(cv2.__version__)
cap = cv2.VideoCapture(0)
if not (cap.isOpened()):
print("Could not open video device")
while(True):
Capture frame-by-frame
ret, frame = cap.read()
Display the resulting frame
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```