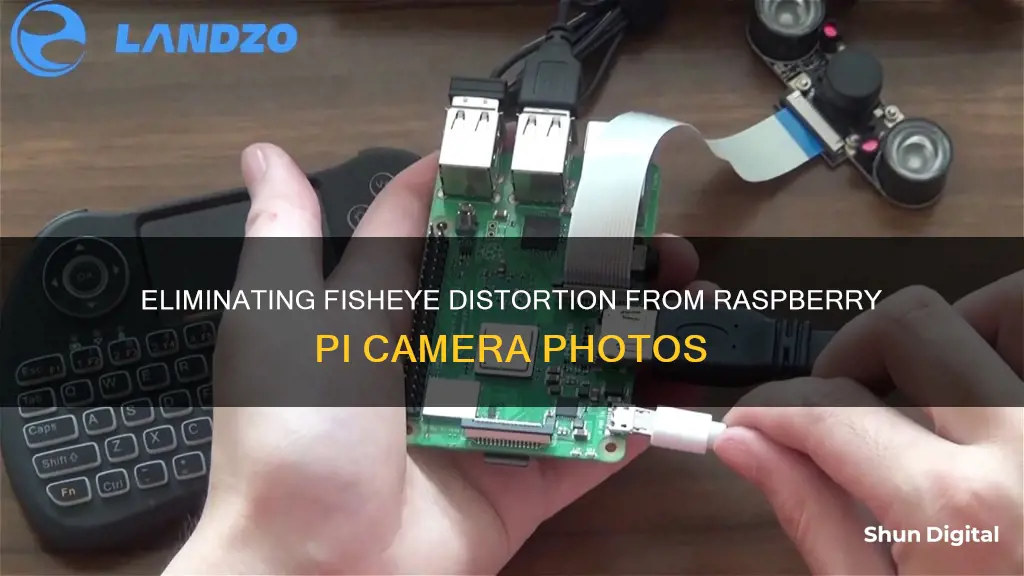
The Raspberry Pi camera is a popular choice for developers and hobbyists due to its versatility and affordability. However, one common issue that users encounter is the fisheye effect, which can distort images and videos. In this guide, we will explore effective methods to remove the fisheye distortion and achieve a more natural-looking output. We will delve into camera calibration techniques, sensor mode adjustments, and the use of different lenses to mitigate the fisheye effect. By following these steps, Raspberry Pi users can capture high-quality visuals without the unwanted curvature and warping typically associated with fisheye lenses.
What You'll Learn
Use OpenCV to calibrate the camera and remove lens distortion
To remove the fisheye effect from a Raspberry Pi camera, you can use OpenCV to calibrate the camera and correct lens distortion. Here's a step-by-step guide:
- Install OpenCV: Ensure you have OpenCV installed on your machine. If you're using Anaconda, you can run the command `conda install -c conda-forge opencv`. Alternatively, you can use `pip install opencv-python`.
- Understand Distortion: Radial distortion and tangential distortion are the two main types of distortion. Radial distortion causes straight lines to appear curved, and it becomes more pronounced the farther points are from the image's centre. Tangential distortion occurs when the camera lens is not perfectly parallel to the imaging plane, making objects appear closer or farther than they actually are.
- Prepare a Chessboard: Chessboard calibration is a standard technique for camera calibration. You can download and print the official chessboard from the OpenCV documentation. This technique is used because a chessboard is flat, has clear corners and points, and all points and corners lie on straight lines, making it ideal for mapping 3D points to 2D camera coordinates.
- Capture Chessboard Images: Take at least 10 photos of the chessboard from different distances and orientations. Tape the chessboard to a flat, solid object, and ensure the images are saved on your computer.
- Find and Draw Corners: Use the `cv2.findChessboardCorners` function in OpenCV to find and draw the corners on the chessboard images.
- Write Calibration Code: Write Python code to perform camera calibration using the chessboard images. The code should include importing necessary libraries, defining chessboard dimensions, and finding corners using `cv2.findChessboardCorners`. After finding the corners, use `cv2.cornerSubPix` to refine their positions.
- Calibrate the Camera: Utilise the `cv2.calibrateCamera` function to perform camera calibration. This function will return the camera matrix, distortion coefficients, rotation and translation vectors.
- Refine Camera Matrix: Use `cv2.getOptimalNewCameraMatrix` to refine the camera matrix and obtain a rectangular region of interest.
- Undistort Image: Apply the undistortion to the image using `cv2.undistort. You can also use remapping by finding a mapping function from the distorted image to the undistorted image and then using the `cv2.remap` function.
- Save Calibration Parameters: If desired, save the camera calibration parameters using a package like Pickle. The key parameters to save are `mtx`, `dist`, and `optimal_camera_matrix`.
By following these steps and utilising OpenCV, you can effectively calibrate your Raspberry Pi camera and remove lens distortion, including the fisheye effect.
The Camera Cringe: Why Do I Shudder?
You may want to see also
Use a different resolution
The Raspberry Pi camera's default resolution is 1920x1080, and it does not have a fisheye effect. However, reducing the resolution to 368x207, which is the same aspect ratio, introduces a strong fisheye effect.
To address this issue, you can force a specific sensor mode when constructing the camera object in your code. Here's an example of how to do it:
Python
Camera = picamera.PiCamera()
Camera.resolution = (368, 207)
Camera.sensor_mode = "something" # Force the desired sensor mode
Camera.start_preview()
By forcing the sensor mode, you can control how the camera captures the image and potentially reduce or eliminate the fisheye effect. The specific sensor mode you should use will depend on your camera model and the desired resolution. You can refer to the picamera documentation to find the appropriate sensor modes for your camera.
Additionally, you can explore other resolutions that may be compatible with your use case and see if they introduce less fisheye distortion. For example, you could try a resolution of 640x480, which is commonly used for security cameras, and see if it provides a better result.
Another approach is to capture images at a higher resolution and then downsize them using image processing libraries like PIL (Python Imaging Library). While this may result in sub-optimal performance, it gives you more control over the final image size and may help reduce the fisheye effect.
It's worth noting that the Raspberry Pi camera modules have improved over time, with the latest Camera Module 3 offering a 12-megapixel resolution. The Camera Module 3 also comes in a standard or wide FoV model, providing additional flexibility. If upgrading your camera module is an option, consider choosing the latest model to take advantage of improved image quality and potentially reduce the fisheye effect.
Viewing Roku's Camera Feed on Your TV
You may want to see also
Use a different lens
If you're looking to remove the fisheye effect from your Raspberry Pi camera, one solution is to use a different lens. Here are some detailed instructions and considerations to help you achieve that:
- Lens Options: The Raspberry Pi camera typically uses a wide-angle or fisheye lens, which can introduce distortion, especially at lower resolutions. To eliminate this effect, you can opt for a different type of lens, such as a standard or telephoto lens. These lenses have narrower fields of view, reducing the amount of distortion in your images.
- Selecting a Lens: When choosing a replacement lens, consider the sensor size of your Raspberry Pi camera. The Raspberry Pi High Quality Camera, for example, uses a 1/2.3" sensor. Make sure the lens you select is compatible with this sensor size.
- Lens Mounts: Pay attention to the lens mount type as well. The Raspberry Pi High Quality Camera uses an M12 mount, which is lightweight, cost-effective, and highly customizable. This type of mount offers a wide range of lenses to choose from, allowing you to find the perfect lens for your specific needs.
- Field of View: Consider the field of view (FoV) that you require for your application. Fisheye lenses typically offer a much wider FoV than standard lenses. If you still want a wide FoV without the distortion, look for a lens with a smaller FoV than a fisheye but larger than that of a standard lens.
- Resolution and Sensor Modes: Keep in mind that the fisheye effect can be influenced by the camera's resolution and sensor mode. As mentioned in your query, reducing the resolution can sometimes introduce a strong fisheye effect. Refer to the picamera docs to understand how different sensor modes and resolutions affect the field of view and distortion.
- Calibration: Proper camera calibration is crucial to reducing lens distortion. This process involves using a calibration pattern, such as a chessboard, to correct for lens distortion. OpenCV (Open Source Computer Vision Library) provides useful tutorials on camera calibration, which can help you eliminate the fisheye effect from your images.
- Post-Processing: If you prefer not to replace the lens, you can also use post-processing techniques to remove the fisheye effect. This involves capturing images at a higher resolution and then reducing the size using software like PIL (Python Imaging Library). However, this method may result in sub-optimal performance, and the image processing may introduce other artefacts.
Remember that using a different lens will not only remove the fisheye effect but also alter the overall look and feel of your images. Consider the aesthetic you wish to achieve and select a lens that suits your creative vision.
Unlocking External Camera Views: A Comprehensive Guide
You may want to see also
Use a different sensor mode
If you are using a fisheye lens with your Raspberry Pi camera, you may notice a fisheye effect when reducing the resolution of your images or videos. This effect can be minimised by adjusting the sensor mode.
The Raspberry Pi camera module offers different sensor modes, which can be selected to optimise for different resolutions and fields of view. The sensor mode can be forced on construction with the sensor_mode parameter.
For example, if you are using the picamera library in Python, you can specify the sensor mode when creating a new camera object:
Python
Camera = picamera.PiCamera(sensor_mode=
By choosing the appropriate sensor mode, you can capture images or videos at your desired resolution without introducing a strong fisheye effect.
It is worth noting that the available sensor modes and their specific characteristics may vary depending on the version of the Raspberry Pi camera module and the software or library you are using. Therefore, it is recommended to refer to the official documentation or community forums for the specific sensor modes supported by your setup.
Removing the VVTi Camera Gear: Step-by-Step Guide
You may want to see also
Use a different camera
If you are experiencing a fisheye effect with your Raspberry Pi camera, one solution is to use a different camera altogether. Here are some alternative camera options that can help you avoid the fisheye distortion:
Choose a Camera with a Rectilinear Lens
Rectilinear lenses are designed to correct the distortion caused by the curved image sensor, resulting in straight lines and a more accurate representation of the scene. Look for cameras that specifically mention "rectilinear lens" or "no fisheye effect" in their specifications. These cameras will provide a wider field of view without the curvature associated with fisheye lenses.
Opt for a Camera with a Higher Resolution
In some cases, the fisheye effect can be more prominent at lower resolutions. If you require a lower resolution image, consider capturing the image at a higher resolution and then downsizing it using appropriate software. This approach may require additional processing power and time but can help mitigate the fisheye distortion.
Explore Different Sensor Modes
The Raspberry Pi camera has different sensor modes that can affect the field of view. At higher resolutions like 1080p, the camera may automatically select a sensor mode with a partial field of view, reducing the fisheye effect. Experiment with different sensor modes to find the one that provides the desired field of view without introducing distortion.
Consider a Camera with a Smaller Field of View
Fisheye lenses are known for their ultra-wide field of view, often exceeding 160 degrees. If the ultra-wide field of view is not a requirement for your project, consider choosing a camera with a smaller field of view, typically around 72 degrees for normal cameras. This narrower field of view will inherently avoid the fisheye effect.
Action Cameras
If you are specifically looking for an action camera without the fisheye effect, there are options available in the market. These cameras are designed to capture fast-paced activities without the distortion commonly associated with fisheye lenses. Check reviews and specifications to find the right action camera for your needs.
Remember, when choosing an alternative camera, consider factors such as compatibility with the Raspberry Pi, image quality, ease of use, and the specific requirements of your project. By selecting a camera with the right features and specifications, you can effectively avoid the fisheye effect and achieve the desired visual outcome.
A Simple Guide to Viewing IPUX Cameras
You may want to see also
Frequently asked questions
You can use the OpenCV calibrateCamera() function to remove lens distortion.
This depends on your specific needs. If you are looking for a fisheye lens, the Arducam 180-degree M12 lens is a good option. If you want a non-fisheye lens, you can try reducing the resolution of your camera or using a different sensor mode.
You can try using the picamera module in Python to set the resolution and sensor mode. For example, you can use camera = picamera.PiCamera() camera.resolution = (368,207) camera.start_preview().
The Raspberry Pi HQ camera has a higher resolution sensor (1/2.3") and can capture images with a wider field of view than the regular Raspberry Pi camera.