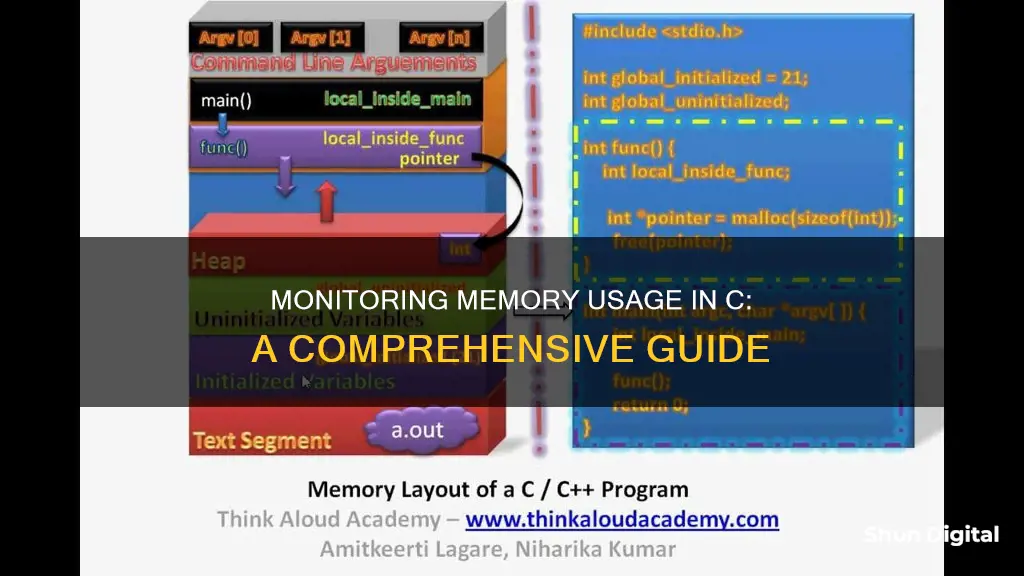
Memory management is a crucial aspect of programming, and developers often need to monitor memory usage to optimise their code and prevent issues like memory leaks. While it's relatively easy to obtain memory usage statistics in Linux, pinpointing the exact memory usage of a specific process can be challenging. This is where tools and techniques for monitoring memory usage in C come into play. One method involves using header files to track memory usage, with malloced_memory_usage keeping tabs on allocated memory in C and memory_usage serving the same purpose in C++. Other tools like Valgrind, GNU time, and POSIX getrusage can also help developers profile memory usage and identify potential memory leaks in their C programs.
Characteristics | Values |
---|---|
Memory usage monitoring tools | Valgrind, Massif, GNU time, POSIX getrusage, Custom Invocation |
Memory usage tracking methods | Calculating the difference in memory before and after allocation, Using header files, Using malloced_memory_usage global variable |
Memory usage statistics | Virtual memory size, Resident set size, RSS, Stack usage, Executable code size, Heap memory |
What You'll Learn
Using Valgrind to monitor memory leaks
Valgrind is a multipurpose code profiling and memory debugging tool for Linux on the x86 and AMD64 architectures. It allows you to run your program in its own environment, monitoring memory usage such as calls to malloc and free (or new and delete in C++). Valgrind can detect if you use uninitialised memory, write off the end of an array, or forget to free a pointer.
To use Valgrind, you must first install it. You can do this by checking if you have it installed and, if not, using the following commands:
Sudo apt install valgrind # Ubuntu, Debian, etc.
Sudo yum install valgrind # RHEL, CentOS, Fedora, etc.
Sudo pacman -Syu valgrind # Arch, Manjaro, Garuda, etc.
Sudo pkg ins valgrind # FreeBSD
Then, to run Valgrind, pass the executable as an argument (along with any parameters to the program). Here is an example:
Valgrind --leak-check=full \ --show-leak-kinds=all \ --track-origins=yes \ --verbose \ --log-file=valgrind-out.txt \ ./executable exampleParam1
The flags used in the example are:
- ```--leak-check=full```: Each individual leak will be shown in detail.
- ```--show-leak-kinds=all```: Show all "definite, indirect, possible, reachable" leak kinds in the "full" report.
- ```--track-origins=yes```: Favour useful output over speed. This tracks the origins of uninitialised values, which could be very useful for memory errors. Consider turning off if Valgrind is unacceptably slow.
- ```--verbose```: Can tell you about unusual behaviour of your program. Repeat for more verbosity.
- ```--log-file```: Write to a file. Useful when output exceeds terminal space.
Valgrind will then produce a report. If there are no leaks, the report will look something like this:
HEAP SUMMARY: in use at exit: 0 bytes in 0 blocks total heap usage: 636 allocs, 636 frees, 25,393 bytes allocated All heap blocks were freed -- no leaks are possible ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0) ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
If there is a leak, the report will show something like this:
5 bytes in 1 blocks are definitely lost in loss record 1 of 1 at 0x4C29BE3: malloc (vg_replace_malloc.c:299) by 0x40053E: main (in /home/Peri461/Documents/executable)
This report is not very helpful as it does not show exactly where the leak is. To get a more detailed report, you can recompile your program with a debug flag (using gcc):
Gcc -o executable -std=c11 -Wall main.c # suppose it was this at first
Gcc -o executable -std=c11 -Wall -ggdb3 main.c # add -ggdb3 to it
Now, Valgrind will point to the exact line of code allocating the memory that got leaked.
There are also some general tips for finding and avoiding memory leaks:
- Use RAII and most of your problems will go away.
- Make sure your dynamically allocated memory gets freed.
- Don't allocate memory and forget to assign the pointer.
- Don't overwrite a pointer with a new one unless the old memory is freed.
Monitoring Data Usage on Android: Tips and Tricks
You may want to see also
Calculating memory usage with POSIX getrusage
To calculate memory usage with POSIX getrusage, you can use the following code:
C
#include
#include
Int main() {
Struct rusage r_usage;
Getrusage(RUSAGE_SELF, &r_usage);
Printf("Memory usage: %ld kilobytes\n", r_usage.ru_maxrss);
Return 0;
}
This code includes the necessary header file, `sys/resource.h`, which defines the `getrusage` function and the `rusage` structure. In the `main` function, a `rusage` structure named `r_usage` is declared. The `getrusage` function is then called with RUSAGE_SELF as the first argument, indicating that information about the current process is requested, and the address of r_usage as the second argument, where the memory usage information will be stored. Finally, the maximum resident set size used by the process, in kilobytes, is printed using `printf`.
Note that the availability and accuracy of memory usage information through `getrusage` may vary across different Linux distributions and kernel versions. Some fields in the `rusage` structure may be unmaintained or always return zero on certain systems.
C
#include
#include
#include
Int main() {
Struct rusage memory;
Errno = 0;
Getrusage(RUSAGE_SELF, &memory);
If (errno == EFAULT) {
Printf("Error: EFAULT\n");
} else if (errno == EINVAL) {
Printf("Error: EINVAL\n");
}
Printf("Usage: %ld\n", memory.ru_ixrss);
Printf("Usage: %ld\n", memory.ru_isrss);
Printf("Usage: %ld\n", memory.ru_idrss);
Printf("Max: %ld\n", memory.ru_maxrss);
Return 0;
}
This code adds error handling by checking the value of `errno` after calling `getrusage`. If `errno` is set to EFAULT, it indicates an error related to invalid memory access. If `errno` is set to EINVAL, it means that an invalid argument was passed to `getrusage`. The memory usage values are then printed using `printf`.
It's important to note that the `getrusage` function provides a snapshot of memory usage at the time it is called. If you want to track memory usage over time or detect memory leaks, you may need to call `getrusage` periodically and calculate the differences in memory usage.
Additionally, you can also use other methods to obtain memory usage information on Linux, such as reading files in the /proc directory or using external tools like valgrind and massif.
Monitoring JVM Heap Usage: Practical Tips for Performance Optimization
You may want to see also
Using the time command to check memory usage
The time command can be used to check the memory usage of a program. The command has a similar syntax to the time command and is simple to use.
To check the memory usage of a program, use the following command:
Command time -v myprogram
This will provide various statistics, including user time, system time, percent of CPU, and maximum resident set size. The resident set size is the amount of memory that the program is using.
It's worth noting that the time command is specific to GNU, and may not be available on all systems. As an alternative, the zsh shell has a built-in time command that can report memory statistics. By setting the TIMEFMT environment variable, you can customise the output to include memory usage information.
For example:
If [[ `uname` == Darwin ]]; then
MAX_MEMORY_UNITS=KB
Else
MAX_MEMORY_UNITS=MB
Fi
TIMEFMT='%J %U user %S system %P cpu %*E total'$'\n'\
'avg shared (code): %X KB'$'\n'\
'avg unshared (data/stack): %D KB'$'\n'\
'total (sum): %K KB'$'\n'\
'max memory: %M '$MAX_MEMORY_UNITS''$'\n'\
'page faults from disk: %F'$'\n'\
'other page faults: %R'
This will provide information such as the average shared and unshared memory, total memory, and maximum memory used by the program.
Monitoring Employee Internet Usage: What Employers Can and Can't Do
You may want to see also
Using Massif to analyse heap memory
Massif is a heap profiler, which means it measures how much heap memory a program uses. It can provide information about heap administration blocks and stack sizes. This can be useful in helping you reduce the amount of memory your program uses, which can, in turn, speed up your program.
To use Massif, specify --tool=massif on the Valgrind command line. The program will then execute, and upon completion, Massif will print summary space statistics. It also creates a graph representing the program's heap usage in a file called massif.pid.ps, which can be read by any PostScript viewer, such as Ghostview.
Massif can also provide details about which parts of your program are responsible for allocating heap memory. This can be useful in identifying where and when your application allocates memory on the heap, with a view to either reducing the amount of memory that you allocate or freeing it sooner.
Monitor Internet Usage: Bloom Broadband's Comprehensive Solution
You may want to see also
Using Custom Invocation on Codeforces to find memory usage
When participating in programming contests on Codeforces, programmers can use Custom Invocation to find out how much memory their code is taking on the contest's server. This is particularly useful when checking for time limits, as you cannot know how fast your code will run on the target machine.
Custom Invocation is especially handy when you have partial feedback, as you can test your code on your local machine, see that it runs in a reasonable time, submit it, pass the pretests, and then get a "time limit" verdict on the main tests.
If you're using static arrays, you can use Custom Invocation to calculate memory usage by adding up the sizes of each array and then dividing by 1048576.0 to get the size in megabytes. Here is the formula:
Sizeof(array_0) + sizeof(array_1) + sizeof(array_2) + ... + sizeof(array_k)) / 1048576.0
This works because the sizeof(a) function returns the size of array a in bytes, so dividing by 1048576 (which is 2^20) converts it into megabytes.
Additionally, if you're on Linux, you can use POSIX getrusage to find out the memory usage of your program. Here is the code snippet:
#include
#include
Int getrusage(int who, struct rusage *usage);
The relevant elements of the struct are ru_idrss and ru_isrss, which stand for integral unshared data size and integral unshared stack size, respectively.
Another option for Linux users is to use the terminal program /usr/bin/time with the -v flag. Here is an example command:
/usr/bin/time -v ./a.out
This will display information such as user time, system time, percent of CPU used, and, most importantly, the maximum resident set size, which is the maximum memory usage of your program.
It's important to note that Custom Invocation on Codeforces is limited by a 256 KB input file size limit, which can be problematic when checking for time limits, as it often involves large test cases.
In conclusion, Custom Invocation on Codeforces is a valuable tool for programmers to assess their code's memory usage and time limits, especially when combined with POSIX getrusage or the /usr/bin/time terminal program on Linux.
Monitoring Android CPU Usage: A Comprehensive Guide
You may want to see also
Frequently asked questions
There are a few ways to monitor memory usage in C. You can use tools like Valgrind, Massif, GNU time, POSIX getrusage, and more. Alternatively, you can calculate memory usage manually by keeping track of malloc, free, and calloc.
Valgrind is a tool that can profile detailed memory usage of your C program and detect memory access violations. It includes specific analysis tools like memcheck and massif for in-depth memory analysis.
Massif is a Valgrind tool that provides heap memory analysis. It can help identify memory leaks and track down allocations of large amounts of memory.
The GNU time command can be used to calculate the memory consumed by a C program after it has finished executing. The command is as follows:
```bash
command time -v myprogram
```
You can include header files like "cmemcounter.h" to help track memory usage in C. By using malloc, free, and calloc as you normally would, the "malloced_memory_usage" global variable will keep track of the allocated memory. However, this method may not intercept all memory allocations and might underestimate usage.