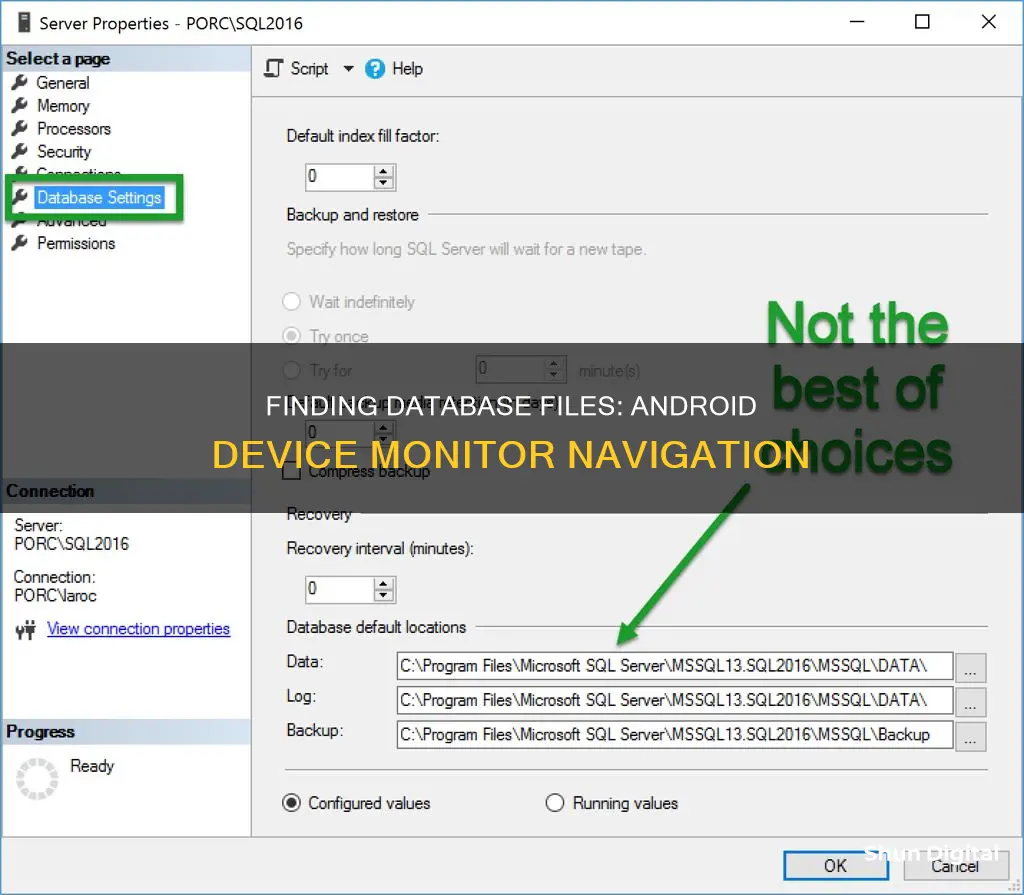
To find a database file in Android Device Monitor, you can follow these steps:
- Open Android Device Monitor via Tools > Android > Android Device Monitor.
- Click on your device on the left-hand side.
- Go to the File Explorer tab (one of the tabs on the right).
- Navigate to /data/data/databases.
- Select the database by clicking on it.
- Click on the 'pull a file from the device' button in the top-right corner of the Android Device Monitor window.
- Choose where you want to save your database file.
Characteristics | Values |
---|---|
Method | Without Opening DDMS |
Steps | Note down the path of the database file in your system, pull the database file into the PC |
If permission is denied, run adb root and run the previous command again | |
Method | Using Stetho library |
Steps | Add Stello dependency in build.gradle, put the following command on the OnCreate() method of the main activity, connect a device and run the app, visit the following site using Chrome browser |
Method | Using SQLiteBrowser |
Steps | Download and install SQLiteBrowser, copy the database from the device to the PC, open DDMS via Tools > Android > Android Device Monitor, click on the device, go to File Explorer, go to /data/data/databases, select the database, click on the ‘pull a file from the device’ button, save the files |
For Android studio >= 3.0, use View > Tool Windows > Device File Explorer to Open device file explorer, go to data/data/PACKAGE_NAME/database, right-click on the database and save it | |
Method | Using ADB shell to connect to Sqlite3 |
Steps | Go to the tools folder in the command prompt, use the command adb devices to see the list of all devices, connect a shell to the device, go to the file containing the DB file, execute sqlite3 to connect to DB, run SQL commands to see any table |
Method | Using Database Inspector |
Steps | Connect a device running on API level 26 or higher, the database schemas appear and you can select the database you want to see |
What You'll Learn
Using ADB shell to connect to Sqlite3
ADB shell can be used to connect to Sqlite3. ADB stands for Android Debug Bridge, and it is a command-line tool that allows communication with an Android device.
- Go to the platform-tools folder in the command prompt: Use the command `adb devices` to get a list of all the connected devices.
- Connect a shell to the device: Use the command `adb -s
shell` to connect a shell to the desired device. - Navigate to the folder containing the database file: Use the `cd` command to navigate to the folder containing the database file. The path is usually `data/data/
/databases/`. - Run Sqlite3 to connect to the database: Use the command `sqlite3
.db` to connect to the database. Replace ` ` with the name of your database file. - Run Sqlite3 commands: Once connected to the database, you can run various Sqlite3 commands to interact with the database. For example, to select all rows from a table, you can use the command `Select * from table1;`.
It is important to note that you may need root access to navigate to the data directory on some devices. In such cases, you can use the `su` command to gain root access before navigating to the database folder.
Additionally, if you encounter any issues with permissions, you can use the `run-as` command to run the ADB shell as a specific user. For example, `run-as
Best Monitor Size for Racing Sim Enthusiasts
You may want to see also
Using Stetho library
Stetho is a tool provided by Facebook that can be used to view the contents of a database file in an Android device. Here's a step-by-step guide on how to use it:
Step 1: Add the Stetho dependency to your app's build.gradle file
Include the following line in the dependencies section:
Compile 'com.facebook.stetho:stetho:1.3.1'
If you are also using Stetho with OkHttp or Retrofit, add the following line as well:
Compile 'com.facebook.stetho:stetho-okhttp3:1.3.1'
Step 2: Initialise Stetho in your Application object
Create a new class that extends the Application class, and in its onCreate() method, add the following line:
Stetho.initializeWithDefaults(this);
Step 3: If using Stetho with OkHttp, add the StethoInterceptor
When constructing the OkHttpClient instance, add the StethoInterceptor as a network interceptor:
OkHttpClient client = new OkHttpClient.Builder()
- AddNetworkInterceptor(new StethoInterceptor())
- Build();
Step 4: Start your emulator or device and open Chrome
Open a new window in Chrome and navigate to chrome://inspect. Your emulator or device should appear in the list. Click on "Inspect" to launch a new window.
Step 5: Inspect your app's database
Click on the "Network" tab to view network traffic in real-time. To inspect your app's SQLite database, go to Resources -> Web SQL. From here, you can view and edit the database.
Note: Stetho does not currently support the Android Async Http Client library.
Monitor Sizes: Understanding the Different Screen Dimensions
You may want to see also
Using SQLiteBrowser
To view the SQLite database on an Android device, you can follow these steps:
- Connect your device and launch the application in debug mode: Ensure that your Android device is connected to your computer, and launch your Android application in debug mode.
- Use adb commands to locate and copy the database file: Use adb commands to navigate to the directory where your SQLite database is stored. You can use the command `adb shell "run-as com.yourpackge.name ls /data/data/com.yourpackge.name/databases/"` to list the database files. Replace `com.yourpackge.name` with your application's package name, which can be found in the manifest file.
- Copy the database file to your SD card: Use the command `adb shell "run-as com.yourpackge.name cat /data/data/com.yourpackge.name/databases/filename.sqlite > /sdcard/filename.sqlite" to copy the database file to your SD card. Replace `filename.sqlite` with the name of your database file.
- Pull the database file to your computer: Use the command `adb pull /sdcard/filename.sqlite to copy the database file from your SD card to your computer. This will place the database file in the same directory as your ADB installation.
- Install SQLiteBrowser: Download and install SQLiteBrowser, a graphical user interface for managing SQLite databases. You can download it from https://sqlitebrowser.org/dl/.
- Open the database file in SQLiteBrowser: Launch SQLiteBrowser and use the "Open Database" option to browse for the database file you copied to your computer. Select the file and open it.
- View the database tables and data: Once the database file is open in SQLiteBrowser, you can explore the database structure, view tables, and browse the data stored in the tables.
By following these steps, you can easily view and manage your SQLite database on an Android device using SQLiteBrowser.
Installing a Rear-View TFT LCD Monitor: A Step-by-Step Guide
You may want to see also
Without opening DDMS
Method 1: Without Opening DDMS
This method works on the Emulator only.
In the first step, note down the path of the database file in your system. For example, let it be
/data/data/com.VVV.file/databases/com.VVV.file.database
Secondly, the database file needs to be pulled into the PC. Use the following command
Adb pull /data/data/com.YYY.module/databases/com.YYY.module.database /Users/PATH/
If it shows permission denied or something similar to it, run adb root and run the previous command again.
Monitoring Hulu Data Usage: Tips to Keep Your Data in Check
You may want to see also
Using Database Inspector
The Database Inspector is a tool in Android Studio that allows you to inspect, query, and modify your app's databases while your app is running. It is available in Android Studio 4.1 and higher.
To open the Database Inspector, go to View > Tool Windows > Database Inspector from the menu bar. Then, run your app on an emulator or connected device running API level 26 or higher. Select the running app process from the dropdown menu. The databases in the currently running app will appear in the Databases pane. Expand the node for the database that you want to inspect.
The Databases pane displays a list of the databases in your app and the tables that each database contains. Double-click a table name to display its data in the inspector window to the right. You can click a column header to sort the data in the inspector window by that column. You can also modify data in a table by double-clicking a cell, typing a new value, and pressing Enter.
If you want the Database Inspector to automatically update the data as you interact with your running app, check the Live updates checkbox at the top of the inspector window. While live updates are enabled, the table in the inspector window becomes read-only and you cannot modify its values. You can also manually update the data by clicking the Refresh table button at the top of the inspector window.
The Database Inspector can run queries against your app's database while the app is running. It can use DAO queries if your app uses Room, but it also supports custom SQL queries. If your app includes more than one database, Android Studio prompts you to select the database to query from a drop-down list. If your query method includes named bind parameters, Android Studio requests values for each parameter before running the query. The query results are displayed in the inspector window.
You can also use the Database Inspector to run custom SQL queries against your app's databases while your app is running. To do this, click Open New Query tab at the top of the Databases pane to open a new tab in the inspector window. Select the database that you want to query from the drop-down list at the top of the New Query tab. Type your custom SQL query into the text field and click Run. The query results that are displayed in the New Query tab are read-only and cannot be modified. However, you can use the custom SQL query field to run modifier statements such as UPDATE, INSERT, or DELETE.
The Database Inspector can only interact with a database while your app maintains a live connection to that database. To prevent database connections from closing, toggle Keep database connections open from off to on at the top of the Databases pane.
G-Sync Monitor: Does the Asus G751J Support It?
You may want to see also
Frequently asked questions
Open the Android Device Monitor and click on your device on the left. Go to the File Explorer tab and navigate to /data/data/databases.
Open the Device File Explorer and go to data > data > PACKAGE_NAME > database. PACKAGE_NAME is the name of your package.
You will only be able to access the database on a physical device if your device is rooted.
In the bottom toolbar, click on 'App Inspection'. Go to data > data > PACKAGE_NAME > database. PACKAGE_NAME is the name of your package.
Select View > Tool Windows > Database Inspector from the menu bar. Select the running app process from the dropdown menu. The database schemas will appear and you can select the database you want to view.