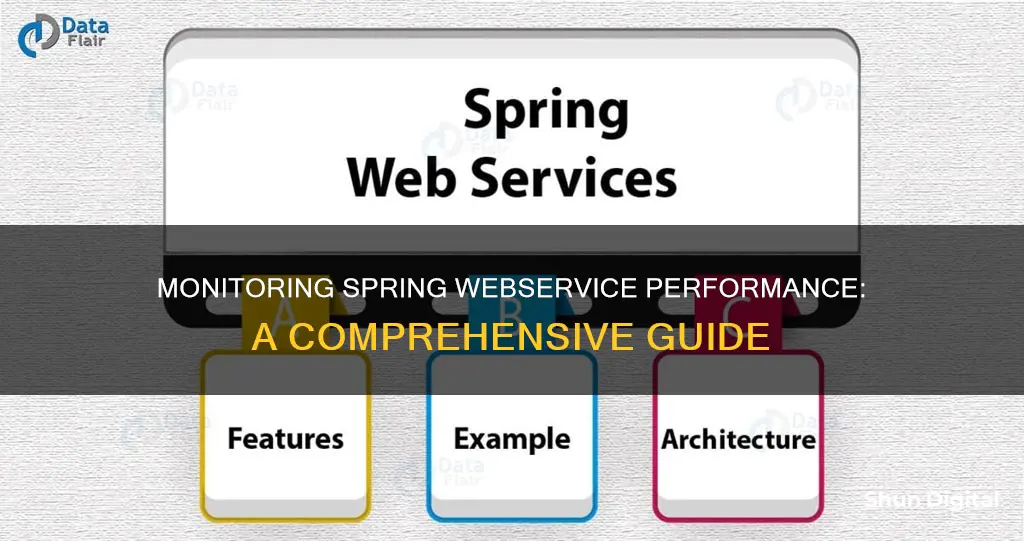
Monitoring the performance of a Spring web service is a critical aspect of software application development and deployment. It ensures that applications are running efficiently and effectively, helping to identify any issues that could negatively impact the user experience. With the increasing complexity of modern applications and the rise of microservices architecture, monitoring performance has become more important than ever.
This article will explore the tools and techniques available to monitor the performance of a Spring web service, providing a comprehensive guide to help developers optimise their applications. We will cover the following topics:
- An overview of Spring Boot performance monitoring and why it is important
- The Spring Boot Actuator and other built-in tools for monitoring Spring Boot applications
- Customising metrics and using Aspect-Oriented Programming (AOP) for more advanced profiling
- An introduction to performance monitoring solutions like Hypersistence Optimizer and FusionReactor APM
Characteristics | Values |
---|---|
Purpose | To ensure Spring Boot applications are running efficiently and effectively, and to help identify any issues that could negatively impact the user experience. |
Importance | Performance monitoring helps identify issues such as slow page load times, high resource usage, and frequent crashes. It also helps identify bottlenecks in the application and provides insights into areas that need improvement. |
Tools | Spring Boot Actuator, Micrometer, Prometheus, Grafana, JProfiler, YourKit, VisualVM, Digma, FusionReactor APM |
Metrics Monitored | Service availability, defect rates, security, business results |
Custom Metrics | Number of logged-in users, currently available stock details, number of orders in the order queue |
What You'll Learn
- Using the PerformanceMonitorInterceptor tool to monitor code performance
- Customising the PerformanceMonitorInterceptor for specific needs
- Using Spring Boot Actuator and Micrometer to collect application metrics
- Identifying and resolving performance issues with Java and Spring Boot profiling tools
- Using FusionReactor APM for real-time monitoring and performance analytics
Using the PerformanceMonitorInterceptor tool to monitor code performance
The PerformanceMonitorInterceptor is a handy tool provided by Spring that helps developers measure and keep track of their code's performance. It serves as a watchful observer, monitoring how fast or slow your code is running and providing valuable insights into your Spring application's performance.
How to Use the Default PerformanceMonitorInterceptor
To use the default PerformanceMonitorInterceptor, you can follow these steps:
- Create a simple class, such as a Person class, and a class with methods that you want to monitor, such as a PersonService class.
- Define a pointcut and advisor by creating an AopConfiguration class with the @Configuration, @EnableAspectJAutoProxy, and @Aspect annotations.
- Within the AopConfiguration class, create a PerformanceMonitorInterceptor bean by returning a new instance of PerformanceMonitorInterceptor(true).
- Create a PerformanceMonitorAdvisor bean by creating an AspectJExpressionPointcut, setting its expression to the desired method, and returning a new DefaultPointcutAdvisor with the pointcut and interceptor.
- Set the log level of the interceptor class to TRACE to see the messages in the console log.
- You can also use logging frameworks like Log4j to achieve the desired log level.
Customizing PerformanceMonitorInterceptor
If you want more control over how performance monitoring is done, you can create your own custom interceptor by extending the AbstractMonitoringInterceptor class. You can override the invokeUnderTrace() method to log the start, end, and duration of a method, as well as set a warning for method executions that last longer than a certain threshold, such as 10 ms.
Benefits of PerformanceMonitorInterceptor
The PerformanceMonitorInterceptor is particularly useful when working on projects with high transaction loads and stringent performance requirements. It helps identify any performance issues early on, even during the development phase. By using this tool, developers can catch and fix performance problems promptly, ensuring their Spring applications meet the required service level agreement (SLA) standards.
H2S Monitors: Who Needs Them and Why?
You may want to see also
Customising the PerformanceMonitorInterceptor for specific needs
The PerformanceMonitorInterceptor is a handy tool provided by Spring that helps developers measure and keep track of their code’s performance. It is customisable to meet specific needs.
To customise the PerformanceMonitorInterceptor, you can extend the AbstractMonitoringInterceptor class and override the invokeUnderTrace() method. This allows you to log the start, end, and duration of a method, as well as set a warning if the method execution takes longer than a specified time. For example, you can log a warning if the method execution lasts more than 10 ms.
Java
Public class MyPerformanceMonitorInterceptor extends AbstractMonitoringInterceptor {
Public MyPerformanceMonitorInterceptor() { }
Public MyPerformanceMonitorInterceptor(boolean useDynamicLogger) {
SetUseDynamicLogger(useDynamicLogger);
}
@Override
Protected Object invokeUnderTrace(MethodInvocation invocation, Log log) throws Throwable {
String name = createInvocationTraceName(invocation);
Long start = System.currentTimeMillis();
Log.info("Method " + name + " execution started at:" + new Date());
Try {
Return invocation.proceed();
} finally {
Long end = System.currentTimeMillis();
Long time = end - start;
Log.info("Method "+name+" execution lasted:"+time+" ms");
Log.info("Method "+name+" execution ended at:"+new Date());
If (time > 10){
Log.warn("Method execution longer than 10 ms!");
}
}
}
In this example, the MyPerformanceMonitorInterceptor class extends AbstractMonitoringInterceptor and overrides the invokeUnderTrace() method. The invokeUnderTrace() method logs the start and end times of a method, as well as the duration of the method execution. If the method execution takes longer than 10 ms, a warning is logged.
You can then associate the custom interceptor with one or more methods by defining a pointcut and creating a bean for the interceptor. Here is an example:
Java
@Pointcut("execution(public int com.baeldung.performancemonitor.PersonService.getAge(..))")
Public void myMonitor() { }
@Bean
Public MyPerformanceMonitorInterceptor myPerformanceMonitorInterceptor() {
Return new MyPerformanceMonitorInterceptor(true);
}
@Bean
Public Advisor myPerformanceMonitorAdvisor() {
AspectJExpressionPointcut pointcut = new AspectJExpressionPointcut();
Pointcut.setExpression("com.baeldung.performancemonitor.AopConfiguration.myMonitor()");
Return new DefaultPointcutAdvisor(pointcut, myPerformanceMonitorInterceptor());
}
In this code, the @Pointcut annotation defines the pointcut expression that identifies the method to be intercepted. The MyPerformanceMonitorInterceptor bean is created and configured with the custom interceptor. The Advisor bean associates the interceptor with the pointcut.
Finally, you can set the log level for the custom interceptor. For example:
Log4j.logger.com.baeldung.performancemonitor.MyPerformanceMonitorInterceptor=INFO, stdout
This will log the output of the custom interceptor to the console.
Breaking Free: Hacking Ankle Monitors for House Arrest
You may want to see also
Using Spring Boot Actuator and Micrometer to collect application metrics
Spring Boot Actuator and Micrometer can be used to collect application metrics. Micrometer is a dimensional-first metrics collection facade that allows developers to time, count, and gauge their code with a vendor-neutral API. Micrometer is included in Spring Boot 2 and has also been backported to Spring Boot 1.5, 1.4, and 1.3.
Micrometer provides a vendor-neutral metrics collection API and implementations for various monitoring systems, including StatsD, Datadog, and Prometheus. Spring Boot auto-configures a composite MeterRegistry and adds a registry for each supported implementation found on the classpath.
To use Micrometer with Spring Boot Actuator, you can follow these steps:
- Add the required dependencies for Micrometer and Spring Boot Actuator to your project.
- Spring Boot will auto-configure a composite MeterRegistry and add registries for supported implementations.
- You can disable specific registries if needed, such as Datadog, by setting the appropriate property in the application properties file.
- You can further configure the MeterRegistry by registering MeterRegistryCustomizer beans to apply common tags or customize individual registry implementations.
- Inject the MeterRegistry into your components and register your custom metrics.
- Spring Boot also provides built-in instrumentation that you can control through configuration or dedicated annotation markers.
- Spring Boot supports various monitoring systems, including Prometheus, Datadog, and New Relic, and provides automatic meter registration for a wide range of technologies.
By following these steps, you can utilize Spring Boot Actuator and Micrometer to collect application metrics and gain insights into the performance of your Spring web service.
LED Monitors: Why You Should Upgrade Your Display
You may want to see also
Identifying and resolving performance issues with Java and Spring Boot profiling tools
Java and Spring Boot profiling tools are essential for identifying and resolving performance issues in your applications. These tools help you analyse runtime behaviour, identify inefficiencies, and optimise your application's performance. Here are some commonly used Java and Spring Boot profiling tools:
JProfiler
JProfiler is a popular choice among developers due to its intuitive user interface. It provides features for visualising system performance, memory usage, potential memory leaks, and thread profiling. JProfiler supports both local and remote applications, allowing you to profile Java applications on remote machines without additional installation. It also offers advanced profiling for SQL and NoSQL databases, making it a versatile tool for various database setups.
YourKit
YourKit is another powerful Java profiler that runs on multiple platforms and provides separate installations for each supported operating system. It offers core features similar to JProfiler, including visualisation of threads, garbage collections, memory usage, and memory leaks. YourKit also supports local and remote profiling via SSH tunnelling. Additionally, its CPU profiling feature allows focused profiling on specific areas of code, such as methods or subtrees in threads.
Java VisualVM
Java VisualVM is a free, open-source, and simplified profiling tool for Java applications. It was previously bundled with the Java Development Kit (JDK) but is now distributed as a standalone tool. Java VisualVM relies on other standalone tools provided in the JDK, such as JConsole, jstat, jstack, jinfo, and jmap. It supports local and remote profiling, memory profiling, and CPU profiling. You can also extend Java VisualVM with new functionalities using plugins, which can be added to its built-in update centre.
NetBeans Profiler
The NetBeans Profiler is bundled with Oracle's open-source NetBeans IDE, providing an all-in-one solution for development and profiling. It shares similarities with Java VisualVM but stands out with its unique feature of tracking garbage collection frequency.
IntelliJ Profiler
IntelliJ Profiler is a simple yet powerful tool for CPU and memory allocation profiling. It combines the capabilities of two popular Java profilers: JFR and Async Profiler. IntelliJ Profiler is easy to use, allowing you to get started with just a few clicks and no complex configuration. It also offers advanced features like differential flame graphs for efficient performance analysis.
Spring Boot Actuator
Spring Boot Actuator is a built-in tool provided by Spring Boot for monitoring and observability. It allows you to expose various endpoints that provide insights into the application's state and performance, including health checks, metrics, and application information. Spring Boot Actuator integrates with monitoring systems like Prometheus and Grafana to provide detailed application metrics.
Hypersistence Optimizer
Hypersistence Optimizer is a performance monitoring tool that can automatically detect JPA and Hibernate performance issues. It helps ensure that your Spring Boot application meets SLA (Service Level Agreement) requirements. Hypersistence Optimizer can be configured to set properties such as timeout thresholds and flush timeouts for the Persistence Context.
PerformanceMonitorInterceptor
PerformanceMonitorInterceptor is a Spring AOP (Aspect Oriented Programming) solution for basic monitoring of method execution times. It uses a StopWatch instance to determine the beginning and ending times of method runs, providing insights into your application's performance.
By leveraging these Java and Spring Boot profiling tools, you can effectively identify and resolve performance issues, optimise your application's efficiency, and enhance the overall user experience.
Choosing the Right Monitor: Size Considerations
You may want to see also
Using FusionReactor APM for real-time monitoring and performance analytics
FusionReactor APM is an all-in-one observability tool with AI insights that helps you monitor your application's performance and health. It provides deep insights into your application, allowing you to detect issues early on and optimize performance.
With FusionReactor, you can identify resource-intensive transactions, monitor the state of installations across all servers, and diagnose issues with your application or server. One of its key features is intelligent alerting, which enables you to identify performance and stability problems instantly.
The tool also offers continuous profiling, allowing you to instantly find and fix performance issues in your applications. This proactive approach helps you address potential problems before they become critical.
Additionally, FusionReactor's advanced anomaly detection capabilities leverage machine learning algorithms to establish baseline performance metrics and quickly identify deviations from normal patterns. This ensures that you maintain peak application performance and that issues are addressed promptly.
FusionReactor APM is a trusted solution, with thousands of companies relying on it to monitor over 35,000 servers. It provides a comprehensive set of tools to help you create advanced websites without wasting time searching for solutions.
Monitoring Java Heap Usage: Tips for Performance Optimization
You may want to see also
Frequently asked questions
The PerformanceMonitorInterceptor is a Spring tool that helps developers measure and track their code's performance. It provides insights into the execution time of methods by using Spring AOP (Aspect Oriented Programming) to intercept method calls.
To use the PerformanceMonitorInterceptor, you need to define a pointcut and advisor. The pointcut expression identifies the methods to be intercepted, and the advisor associates the interceptor with the pointcut. You also need to enable AspectJ support and set the log level of the interceptor class to TRACE.
Yes, you can implement your own custom interceptor by extending the AbstractMonitoringInterceptor class and overriding the invokeUnderTrace() method to log the start, end, and duration of a method, as well as any warnings.
A typical monitoring system for Spring Boot applications would include a dashboard for data visualisation, a metric store (time-series database), and applications that supply the metric store with data from the application's local state. Additional components like alerting via email or Slack are also useful.
You can use a combination of tools like JProfiler, YourKit, VisualVM, Spring Boot Actuator, Micrometer, distributed tracing tools like Zipkin or Elastic APM, and platform-specific tools like Digma. These tools help with CPU and memory usage analysis, garbage collection, database interactions, and more.