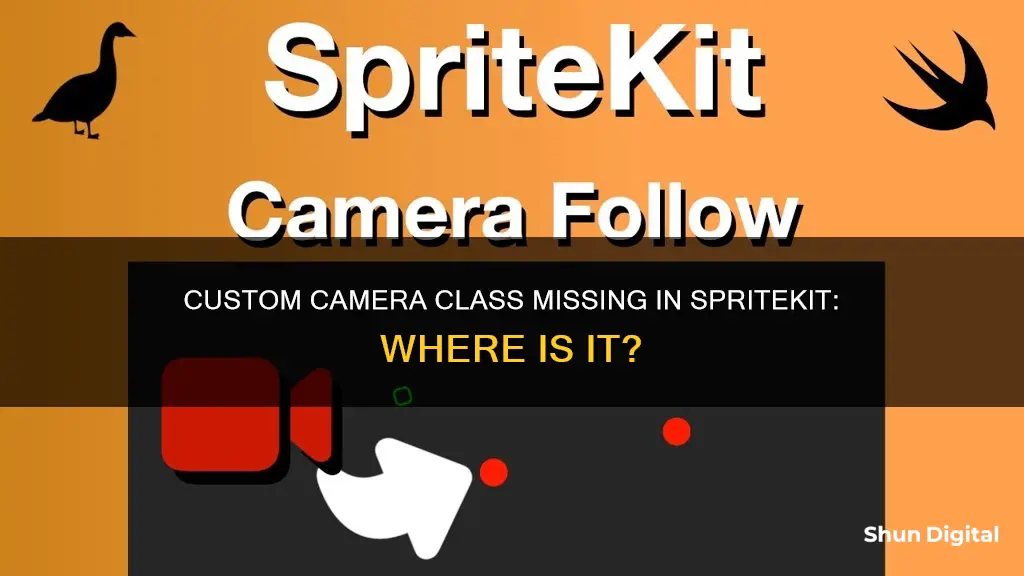
SpriteKit is a powerful 2D sprite-based framework for game development from Apple. When creating a SpriteKit game, developers can use custom classes to define the behaviours of game elements such as the main character. However, in some cases, the custom class may not be recognised or executed as expected, leading to issues in the game's functionality. This can be frustrating for developers, especially when there are no errors indicated. To resolve this, it is important to ensure that the custom class is correctly linked to the .sks file and that the player node is properly assigned to the custom class. Additionally, it is worth checking for any updates or changes in the development environment that may impact the behaviour of custom classes.
Characteristics | Values |
---|---|
Issue | Custom class not working |
Cause | SKS file not linked to the swift file |
Solution | Type MenuScene into the Custom Class field in the Custom Class Inspector |
Custom Class Sprite Kit
When creating a custom class in Sprite Kit, you will need to create a new file for your custom class and define its behaviours there.
In the new file, you will need to import SpriteKit and define the custom class as a subclass of an existing SpriteKit class, such as SKSpriteNode. You can then implement the desired behaviours and methods within this custom class.
For example, let's say you want to create a custom class for your main character. You can create a new file called "Custom.swift" and define the class "Custom" as a subclass of SKSpriteNode:
Swift
Import SpriteKit
Class Custom: SKSpriteNode {
// Custom class properties and methods
}
Now, you can add your custom behaviours and methods inside the "Custom" class. For instance, you could add a method called "didMoveToScene()":
Swift
Import SpriteKit
Class Custom: SKSpriteNode {
Func didMoveToScene() {
Print("It Worked")
}
}
To use this custom class in your SpriteKit scene, you need to
Now, back in your code, you can access and interact with your custom class through this node. For example, if your node is named "player", you can do the following:
Swift
If let customNode = self.childNode(withName: "player") as? Custom {
CustomNode.didMoveToScene()
}
This will
It is important to ensure that you have properly associated the custom class with the node in your .sks file. If the custom class is not linked correctly, the code inside your custom class may not execute as intended.
Ulta's Camera Surveillance: What You Need to Know
You may want to see also
Custom Class file
A custom class file is a file that contains a class definition with custom behaviours and properties. In the context of SpriteKit, a custom class can be used to define the behaviours of a game character or a specific scene.
To create a custom class in SpriteKit, you would typically follow these steps:
- Define the Custom Class: Start by creating a new file specifically for your custom class, separate from your main code file. Give it a descriptive name, such as "CustomClass.swift". In this file, you will define the class and its properties.
- Implement the CustomNodeEvents Protocol: If you want your custom class to interact with other classes or respond to specific events, you can implement the CustomNodeEvents protocol. This protocol allows you to define functions that will be called when certain events occur, such as when the node is added to a scene.
- Create an Instance of the Custom Class: In your main code file, typically the GameScene.swift file, you will create an instance of your custom class. You can then set this instance as the custom class for your game character or any other node you want to customise.
- Connect the Custom Class to the Node: In your GameScene.sks file, select the node you want to customise (e.g., the player character) and set its custom class to the name of your custom class. This associates the custom behaviours and properties with that specific node.
- Call Custom Functions: Now, when you want to trigger a custom function or behaviour, you can call it using the instance of your custom class. For example, if you have a "didMoveToScene" function in your custom class, you can call it using the custom node instance.
CustomClass.swift:
Swift
Import SpriteKit
Class CustomClass: SKSpriteNode, CustomNodeEvents {
Func didMoveToScene() {
Print("Custom behaviour triggered!")
# Add your custom code here
}
}
GameScene.swift:
Swift
Class GameScene: SKScene {
Var player: SKSpriteNode!
Override func didMove(to view: SKView) {
Player = self.childNode(withName: "//player") as! CustomClass
# Now you can call custom functions on the player instance
Player.didMoveToScene()
}
}
GameScene.sks:
- Select the player node in the scene editor.
- In the Custom Class inspector, type "CustomClass" as the custom class for the player node.
By following these steps, you can create a custom class file and associate it with specific nodes in your SpriteKit game, allowing you to define custom behaviours and properties for those nodes.
Unexpected TV Camera Break: Impact and Aftermath
You may want to see also
Custom Class Inspector
The Custom Class Inspector is a feature in Xcode that allows developers to associate a custom class with a .sks file. This is done by opening the right-side panel in Xcode, accessing the Custom Class Inspector, and then typing the name of the custom class into the Custom Class field.
For example, if you have a .sks file named "MenuScene," you would open the Custom Class Inspector and type "MenuScene" into the Custom Class field to link the .sks file to the corresponding .swift file.
This process ensures that the .sks file, which contains the visual design of the scene, is connected to the .swift file, which contains the custom code for that scene. By doing this, you can modify and update the scene's properties and behaviours in the .swift file, and have those changes reflected in the .sks file when the game is run.
It is important to note that simply creating a .sks file and a .swift file with the same name does not automatically link them. The Custom Class Inspector is the tool used to establish this connection, ensuring that your custom code is applied to the correct scene in your SpriteKit game.
When working with SpriteKit, it is common to create custom classes for different scenes, nodes, and behaviours in your game. The Custom Class Inspector is a crucial tool for connecting these custom classes to the visual elements in your game, allowing you to bring your game to life with your own code.
Q-See Cameras: Compatible with Other DVR Systems?
You may want to see also
SKS file
An SKS file is a SpriteKit Scene file. These are not necessary for your project, but they can be useful for interface building. You can create SKScene content directly in Swift code, but SKS files provide a nice game design/production workflow. You can create simple, reusable nodes (e.g. crates, monsters, coins) and use them across your game.
If you want to associate a node you create in your SKS file with a custom class, you need to:
- Add an empty node into your SKS file
- Open the Custom Class inspector in Xcode
- Type the name of your class into the Custom Class field
- Type the name of your project into the Module field
Custom classes are only supported on Xcode 7 and iOS 9. If you are using an earlier version, you will need to use a workaround.
Wyze Camera: Is Someone Spying on You?
You may want to see also
Swift file
In SpriteKit, a Swift file is used to define the behaviour and properties of a custom class. This allows you to create reusable and modular code, making it easier to manage and update your game or application.
Creating the Custom Class: First, you need to create a new Swift file and define your custom class. For example, let's say you want to create a custom class for your main character:
Swift
Import SpriteKit
Class CustomCharacter: SKSpriteNode {
// Add your custom properties and methods here
}
Defining Properties and Methods: Inside your custom class, you can define properties and methods specific to that class. For example:
Swift
Class CustomCharacter: SKSpriteNode {
Var health: Int = 100
Var speed: CGFloat = 5.0
Func moveLeft() {
Position.x -= speed
}
Func moveRight() {
Position.x += speed
}
}
Using the Custom Class in Your Scene: To use your custom class in your SpriteKit scene, you need to create an instance of it and add it to your scene hierarchy:
Swift
Class GameScene: SKScene {
Var player: CustomCharacter!
Override func didMove(to view: SKView) {
Player = CustomCharacter(imageNamed: "player.png")
Player.position = CGPoint(x: 100, y: 100)
AddChild(player)
}
}
- Linking the Swift File to the SKS File: If you are using an .sks file for your scene, you need to link the Swift file to the .sks file. Open the .sks file in Xcode, go to the Custom Class Inspector, and enter the name of your custom class (e.g., "CustomCharacter") in the Custom Class field.
- Initialising and Configuring the Custom Class: You can initialise and configure your custom class instances in the scene's `didMove(to view:` method. You can set their position, size, and other properties as needed.
- Interacting with the Custom Class: You can interact with your custom class instances in your scene by calling their methods or accessing their properties. For example, you can call the `moveLeft()` or `moveRight()` methods on the `player` instance to move it left or right.
By using Swift files to define custom classes, you can create reusable and modular code, making your SpriteKit projects more organised and easier to maintain.
Tracking Hockey: TV Cameras and the Puck
You may want to see also
Frequently asked questions
You can create a custom class for your main character by defining its behaviours in a separate file. Start by creating a protocol and then calling the function in the GameScene class.
To link your .sks file to a custom class, open the right-side panel and the Custom Class Inspector. Type the name of your custom class into the Custom Class field.
Check that you have typed the name of your custom class correctly into the Custom Class Inspector. If it is correct, check that the .sks file is linked to the .swift file.