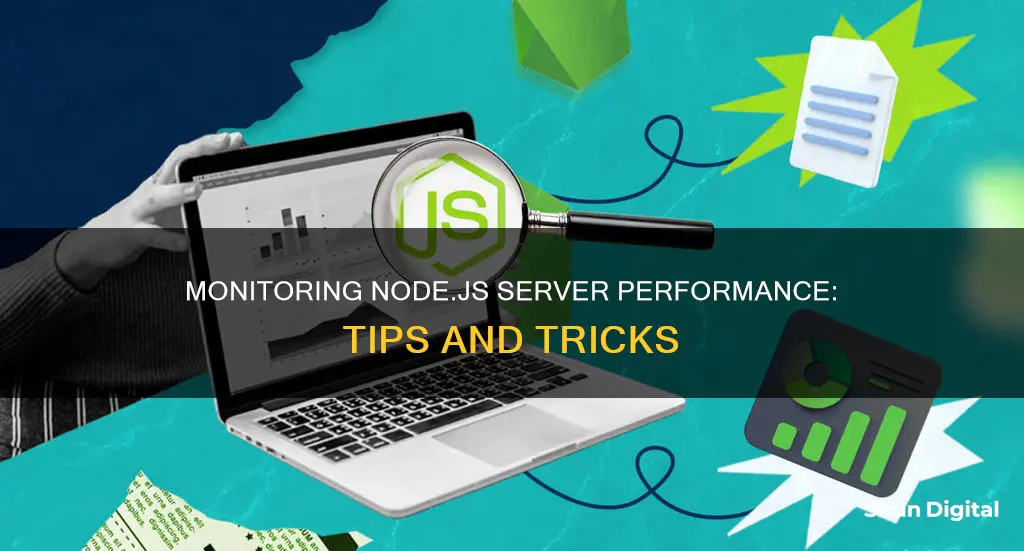
Monitoring Node.js server performance is crucial to ensure optimal application performance and user experience. Node.js applications generate vast amounts of data about their behaviour, and by analysing key metrics, developers can identify performance bottlenecks and potential issues. This includes monitoring CPU usage, memory allocation, and event loop lag to prevent downtimes and ensure efficient resource usage. Additionally, understanding network connections and request handling is essential for optimising response times and maintaining high availability. With the right tools and frameworks, developers can gain valuable insights into their Node.js applications' performance and take proactive measures to improve stability and efficiency.
What You'll Learn
Monitor CPU usage
Monitoring CPU usage is crucial for Node.js applications to ensure optimal performance and resource allocation. Here are some detailed instructions and considerations for monitoring CPU usage:
Understanding CPU Utilization
CPU utilization refers to the amount of CPU time your application requires to handle requests. High CPU usage can be an indicator of inefficient code, excessive requests, or other performance bottlenecks. By monitoring CPU usage, you can identify potential issues and optimize your application's performance.
Tools for Monitoring
There are several tools available for monitoring CPU usage in Node.js applications. Here are some popular options:
- Os-profiler: This is a lightweight utility that provides a snapshot of system resource utilization, including CPU, memory, and disk usage. It is ideal for real-time profiling of system utilization.
- System-health-monitor: This module checks RAM and CPU characteristics and determines if an instance is overloaded based on a configured threshold.
- Process-cpu-utilization: This tool displays the CPU utilization of a process by executing the "ps" shell command.
- ClinicJs: A set of tools that allow you to collect data and display performance charts, including CPU consumption.
- Node.js built-in module: Starting from version 8.x, Node.js provides a native module for CPU profiling, which can be used programmatically without interrupting your application.
Setting Up Alerts and Thresholds
It is essential to set up alerts and thresholds for CPU usage to enable proactive monitoring. You can set alerts based on heartbeat alerts (server downtime), threshold-based alerts (when a certain metric value is crossed), or anomaly-based alerts (detecting patterns in logs that deviate from the baseline). For example, you can set an alert for when the average response time increases above a certain threshold, such as three seconds.
Visualizing CPU Data
Visualizing CPU data can help you identify patterns and anomalies more easily. You can use flame graphs or flame charts to visually represent the collected CPU data. These graphs show the stack profile population on the x-axis and stack depth on the y-axis, with larger boxes indicating higher CPU consumption. Additionally, tools like ClinicJs can generate flame graphs based on CPU consumption.
Best Practices and Considerations
- Baseline Establishment: Collect data for a week or two to establish a baseline for normal CPU usage patterns. This will help you identify deviations and potential issues more effectively.
- Real-time Monitoring: Aim for real-time CPU monitoring solutions to ensure you can react promptly to any issues. Solutions like os-profiler and the Node.js built-in module offer real-time data.
- Proactive Monitoring: Anomaly detection through monitoring can help you identify patterns that may cause future issues. For example, detecting a high number of failed login attempts could indicate unauthorized access attempts.
- Performance Overhead: Keep in mind that CPU profiling tools can impact the performance of your application. Start with short CPU profiling durations and gradually increase them while monitoring the impact on your infrastructure.
- Data Collection and Storage: Consider how you will collect and store CPU profiling data. The Node.js module allows you to collect data programmatically and send it to storage systems like S3.
Inns and Internet Privacy: What You Need to Know
You may want to see also
Monitor memory leaks
Memory leaks can be a serious issue in Node.js applications. They occur when the application allocates memory but fails to release it when it is no longer needed, causing the app to consume more and more memory over time. This can lead to performance issues and even crashes.
To monitor memory leaks in Node.js, you can use various tools and techniques:
- Heap Snapshots: Heap snapshots allow you to see how much memory your application is using and where it is being allocated. By capturing snapshots at different times, you can compare memory usage and identify objects that are not being released, indicating potential memory leaks.
- Profiling Tools: These tools help you identify which parts of your code are using the most memory, allowing you to pinpoint potential memory leaks.
- Logging: By tracking memory usage over time, you can identify trends and patterns that may indicate a memory leak.
- Garbage Collector: Node.js has a built-in garbage collector that can help clean up unused memory. Ensure your code is structured to allow the garbage collector to work effectively.
- Monitoring Tools: Use tools like Sematext Monitoring to gain insights into your application's memory usage and detect memory leaks. These tools provide historical data and alerts when your memory reaches a certain threshold.
- Chrome DevTools: You can use Chrome DevTools to inspect Node.js apps and take snapshots of memory usage.
- Allocation Snapshots: With allocation snapshots, you can view the amount and percentage of memory allocated by each method on the process's call stack, helping to diagnose memory leaks.
By utilizing these tools and techniques, you can effectively monitor memory leaks in your Node.js applications and take appropriate actions to optimize performance and prevent crashes.
TFT vs LCD: Understanding Monitor Technology
You may want to see also
Monitor network connections
Monitoring network connections is crucial when building Node.js applications to ensure optimal performance. Here are some detailed instructions and tools to help you monitor network connections effectively:
Tools and Techniques
- Middleware: Using middleware like Express can help you monitor network traffic. You can replace the require('http') statement with require('./http-log'), where http-log is a wrapper that logs details by binding extra events while returning the original.
- External HTTP Debugging Tools: Tools like node-http-proxy, middlefiddle, mitmproxy, and fiddler can help you monitor network traffic. Route your application's traffic through these tools instead of directly to the server.
- Chrome DevTools: The Chrome DevTools, accessible through the "Network" tab, provide valuable insights into network requests and can assist in debugging network issues.
- Node-inspector: node-inspector is a useful tool for monitoring network traffic, especially if you're using an older version of Node.js (earlier than Node 8).
- Request-debug: If you're using the "request" package, installing the "request-debug" package can help. Simply include require('request-debug')(request) in your code to print all request data to the console.
- Nock Recorder: Nock Recorder logs all HTTP(S) communications to the console. Import nock from 'nock' and use nock.recorder.rec({ output_objects: true }) to start recording.
- Console Logging: You can manually output the contents of response headers within your request call to the console for basic monitoring.
- HTTP Toolkit: HTTP Toolkit is a macOS tool that can be used to intercept Node and Chrome network traffic.
- Debugging-aid: debugging-aid is an npm package that can be used to see outgoing traffic URLs and the associated stack traces.
- Node-network-devtools: This package automatically tracks all HTTP and HTTPS requests from your Node.js application and displays them in a Chrome Network tab-like UI.
Additional Considerations
- Fiddler: While not a direct replacement for Chrome DevTools, Fiddler is a useful tool for monitoring network traffic, especially when working with Windows.
- APM (Application Performance Monitoring): APM tools provide insights into the performance of your Node.js applications. Some examples include Dynatrace and Sentry.
- Trace: Trace is a tool developed by RisingStack specifically for monitoring and debugging Node.js applications. It offers features such as performance measurement, memory heapdump investigation, and CPU profiling.
- HTTP Network Monitor: This is a Node.js-powered tool that captures network packets and provides an admin dashboard for monitoring. It requires Node.js and NPM to be installed and has specific instructions for setting it up on a Raspberry Pi.
Buy Broken Acer S230HL Monitors: Where and How?
You may want to see also
Monitor response times
Monitoring response times is a crucial aspect of ensuring optimal performance and scalability in Node.js applications. It helps identify potential bottlenecks and address performance issues. Here are some detailed instructions and considerations for monitoring response times:
Understanding Response Time Metrics
Firstly, it's important to define what is meant by "response time". In the context of Node.js, response time typically refers to the duration from when the server receives a request to when it generates and sends a response back to the client. This includes the time taken to process the request and send data back to the user.
Tools for Measuring Response Time
There are several tools available to measure response time in Node.js applications:
- Performance Timing API: Node.js provides the Performance Timing API, which is specifically designed for measuring performance. It offers more accurate measurements and handles issues like system time interference.
- Date Object: While not as accurate, you can use the Date object in Node.js to measure the time difference between receiving a request and sending a response.
- Response-time Module: This Node.js module available through the npm registry creates a middleware that records the response time for requests in HTTP servers. It adds an "X-Response-Time" header to responses, allowing you to track the elapsed time.
- N|Solid: N|Solid is a performance monitoring tool that collects runtime-level performance data with minimal overhead on your application. It provides various metrics, including the 99th percentile and HTTP median response times, helping you identify outliers and performance bottlenecks.
- AppDynamics: AppDynamics offers end-to-end Node.js application performance monitoring. It provides features such as automatic discovery of application topology, code-level diagnostics, and performance visualization tools like flame graphs.
Best Practices and Considerations
When monitoring response times, keep the following in mind:
- Server Response Time vs. Connection Latency: Differentiate between server response time and connection latency. Connection latency refers to the time taken for the initial three-way handshake process to complete before data transmission, while server response time focuses on the duration after the request is received.
- Set Baselines and Thresholds: Establish baseline performance metrics for your application and define what constitutes "normal" behaviour. This will help you identify deviations and set appropriate thresholds for alerts.
- Monitor CPU and Heap Usage: In addition to response times, monitor CPU and heap usage. CPU profiles and heap snapshots can help identify issues causing high CPU usage and memory leaks, respectively.
- Real-time Alerting: Implement a real-time alerting system that notifies your operations team when response times slow down or errors occur. Integrate with tools like Pagerduty, Opsgenie, Slack, or Email to ensure prompt notification and response.
Monitor Ghosting: How to Identify and Fix Screen Issues
You may want to see also
Monitor garbage collection
Monitoring garbage collection is crucial for detecting performance degradation in a Node.js application. It involves tracking the Garbage Collection (GC) cycles and analysing the GC logs to identify potential memory issues and memory leaks.
To enable garbage collection traces, you can use the `--trace-gc` flag when running your Node.js application. This flag outputs all garbage collection events in the console, providing information such as the process ID, timestamp of the event, type of GC event, heap usage before and after GC, and the duration of the GC cycle.
By examining the GC logs, you can identify two types of entries: Scavenge and Mark-sweep. Scavenge is an algorithm that performs garbage collection in the "new space" of the heap, where new objects are created. It runs frequently and is responsible for cleaning up small objects. On the other hand, Mark-sweep is a less frequent GC event that occurs in the "old space" of the heap, where objects that survived the Scavenge cycles are moved. It takes longer to identify which objects can be cleaned up and consists of two phases: Mark and Sweep.
When monitoring garbage collection, there are several indications to watch for. Longer GC cycles, for example, can lead to increased latency for application requests. Additionally, tracking the amount of released memory is important. In a healthy application, each GC cycle should release unreachable objects, and the total heap allocated should return to the level of an idle application. If the allocated memory increases along with the application request count and is not released when the request count decreases, it indicates a memory leak that can eventually lead to an application crash.
To identify and address memory leaks effectively, it is crucial to have data and internal insights into how the application manages its memory. Node.js provides a built-in mechanism to output information about the V8 engine's Garbage Collection cycles, allowing you to monitor the frequency, duration, and memory freed up by each GC cycle.
Finding Your Monitor's Password: A Step-by-Step Guide
You may want to see also
Frequently asked questions
A well-performing Node.js application should have minimal or no downtime due to preventable outages, predictable resource usage, and effective scaling to meet peak usage demands.
There are several tools available for monitoring Node.js server performance, including:
- NodeReaction
- Prometheus
- Trace
- PM2
- Better Uptime
- Sematext
- Appmetrics
- Raygun
- Express Status Monitor
- Clinic.js
- AppSignal
- AppDynamics
- Grafana
Some common problems with Node.js applications include service downtimes, slow services and terrible response times, memory leaks, and errors in the code. To fix these issues, you can use a Node.js monitoring tool that can help with detecting and preventing downtimes, collecting data on process levels, requesting CPU profiles, and collecting memory usage data to identify and fix memory leaks.