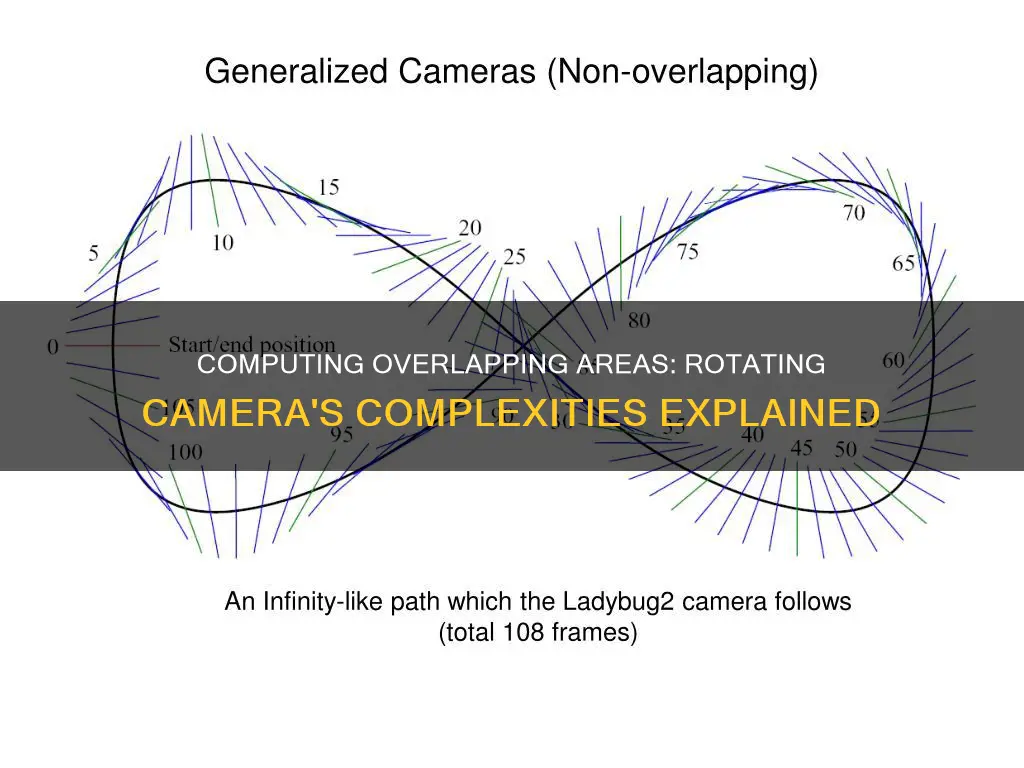
When working with cameras, it is often necessary to understand how to calculate the overlapping area when rotating the camera. This is particularly relevant in fields such as computer vision, robotics, and panoramic photography. To compute the overlapping ratio of two rotated rectangles, one can use the Sutherland-Hodgman polygon clipping algorithm or other methods that involve finding feature points and matching them across images. The specific approach depends on factors such as the number of cameras, their arrangement, and the nature of the scene being captured.
Characteristics | Values |
---|---|
Problem | Given two rectangles, and we know the position of four corners, widths, heights, angles. How to compute the overlapping area of these two rectangles? |
Solution 1 | Draw and overlay the rectangles in 2 different colours (e.g. red and blue) and calculate the number of pixels of the mixed colour (e.g. purple) |
Solution 2 | Sutherland-Hodgman polygon clipping algorithm. Clip one of the polygons with the four supporting lines (half-planes) of the other. Find the intersection polygon and use the polygon area formula to find the area. |
Solution 3 | Rotate both rectangles around the same point so that one of them becomes axis parallel. Then, use the RotatedRectangleIntersection function to get the contour and use the contourArea function to get the area. |
What You'll Learn
Utilise the Sutherland-Hodgman polygon clipping algorithm
The Sutherland-Hodgman algorithm is a widely used algorithm for polygon clipping. It was developed by Ivan Sutherland and Gary Hodgman in 1974 and is used to clip each edge of a polygon against each edge of a boundary in a clockwise order. The algorithm is simple and straightforward to implement and can handle convex and concave polygons, as well as polygons with holes and self-intersections.
The algorithm begins with an input list of all vertices in the subject polygon. Next, one side of the clip polygon is extended infinitely in both directions, and the path of the subject polygon is traversed. Vertices from the input list are inserted into an output list if they lie on the visible side of the extended clip polygon line, and new vertices are added to the output list where the subject polygon path crosses the extended clip polygon line.
This process is repeated iteratively for each clip polygon side, using the output list from one stage as the input list for the next. The four possible situations while processing are:
- If the first vertex is outside the window and the second vertex is inside the window, then the second vertex is added to the output list. The point of intersection of the window boundary and polygon side (edge) is also added to the output list.
- If both vertices are inside the window boundary, then only the second vertex is added to the output list.
- If the first vertex is inside the window and the second vertex is outside the window, then the edge which intersects with the window is added to the output list.
- If both vertices are outside the window, then nothing is added to the output list.
The Sutherland-Hodgman algorithm can be extended into 3D space by clipping the polygon paths based on the boundaries of planes defined by the viewing space. However, it has some drawbacks, such as being inefficient and slow for large or complex polygons due to processing every edge of the polygon against every edge of the boundary. It can also generate degenerate polygons if the polygon is completely outside or inside the boundary and can lead to numerical errors and rounding issues.
The Evolution of Disc Camera: A Historical Perspective
You may want to see also
Rotate polygons around the origin
To rotate a polygon around the origin, you need to apply a rotation matrix to the polygon's vertices. This involves performing some matrix operations on the coordinates of the polygon's points.
Firstly, let's define the problem. Given a polygon with vertices (points) $ [ (x_1, y_1), (x_2, y_2), ..., (x_n, y_n) ]$, we want to find the new coordinates of these points after rotating the polygon by an angle $\theta$ around the origin.
The formula for rotating a point $(x, y)$ around the origin by an angle $\theta$ is given by:
$$X' = \cos(\theta) \cdot X - \sin(\theta) \cdot Y$$
$$Y' = \sin(\theta) \cdot X + \cos(\theta) \cdot Y$$
Where $X'$ and $Y'$ are the new $X$ and $Y$ coordinates of the point after rotation.
Now, to rotate the entire polygon, we need to apply this transformation to each vertex of the polygon. This can be done by creating two arrays, one for the $X$ coordinates and one for the $Y$ coordinates, and then performing matrix operations on these arrays.
- Create two arrays, $X$ and $Y$, of length $n$ (the number of vertices in the polygon). Populate these arrays with the $X$ and $Y$ coordinates of the polygon's vertices, respectively.
- Compute the rotation matrix $R$ for the angle $\theta$:
$$R = \begin{bmatrix} \cos{\theta} & - \sin{\theta} \\ \sin{\theta} & \cos{\theta} \\ \end{bmatrix}$$
Multiply the $X$ and $Y$ arrays by the rotation matrix $R$:
$$X_{new} = R \cdot X$$
$$Y_{new} = R \cdot Y$$
Where $X_{new}$ and $Y_{new}$ are the new arrays of $X$ and $Y$ coordinates after rotation.
Combine the new $X$ and $Y$ coordinates to get the final coordinates of the rotated polygon:
$$P_{new} = \begin{bmatrix} X_{new} \\ Y_{new} \end{bmatrix}$$
$P_{new}$ now contains the coordinates of the vertices of the polygon after rotating it by an angle $\theta$ around the origin.
Note that this method assumes you are working in a 2D coordinate system. If you are working in 3D, you would need to use a 3D rotation matrix and include the $Z$ coordinates in your calculations.
Traffic School: Camera Tickets, What You Need to Know
You may want to see also
Use OpenCV's RotatedRectangleIntersection function
To compute the overlapping area when rotating the camera, you can use OpenCV's RotatedRectangleIntersection function. This function takes two RotatedRect objects and an optional OutputArray parameter. It returns the vertices of the intersecting region if there is any overlap between the two rectangles. Here's an example code snippet in Python:
Python
Import cv2
Define the two rotated rectangles
Rect1 = cv2.RotatedRect(center=(100, 100), size=(50, 50), angle=45)
Rect2 = cv2.RotatedRect(center=(150, 150), size=(50, 50), angle=30)
Find the intersection
IntersectingRegion = cv2.RotatedRectangleIntersection(rect1, rect2)
Check if there is an intersection
If intersectingRegion is not None:
Process the overlapping area
Print("There is an overlap between the two rectangles")
You can now compute the overlapping area using the vertices of the intersectingRegion
Else:
Print("There is no overlap between the two rectangles")
In this example, we first define two RotatedRect objects, rect1 and rect2, by specifying their center, size, and rotation angle. We then call the cv2.RotatedRectangleIntersection function, passing these two rectangles as arguments. The function returns the vertices of the intersecting region, which we store in the intersectingRegion variable.
We then check if the intersectingRegion is not None, which indicates that there is an overlap between the two rectangles. If there is an overlap, you can proceed to compute the overlapping area using the vertices of the intersectingRegion. If there is no overlap, you can handle that scenario accordingly.
Note that the RotatedRectangleIntersection function may not work correctly when the two rectangles are very similar or almost completely overlap. In such cases, you may need to adjust the rectangles slightly or consider using alternative methods to compute the overlapping area.
Additionally, when using the RotatedRectangleIntersection function, make sure that the rotation of both rectangles is done around the same point. If the rotations are performed around different points, the result may be incorrect.
Traffic Tickets: Portland's Camera Enforcement Strategy
You may want to see also
Calculate the intersection area of two rectangles
When dealing with the problem of computing the overlapping area when rotating a camera, one specific case is calculating the intersection area of two non-rotated rectangles. This is a simpler problem that does not involve the added complexity of rotation.
To calculate the intersection area of two non-rotated rectangles, you can start by defining each rectangle using two point tuples {x1, x2, y1, y2}, representing the coordinates of the rectangle. The goal is to find the area of overlap between these two rectangles.
The first step is to find the bounding rectangles of each rectangle. The bounding rectangle of a rectangle is the smallest rectangle that encloses it. You can calculate the left, right, top, and bottom coordinates of each bounding rectangle using the following formulas:
- Left = x1
- Right = x1 + x2
- Top = y1
- Bottom = y1 + y2
Once you have the bounding rectangles, you can calculate the overlap area using the following formulas:
- X_overlap = max(0, min(rect1.right, rect2.right) - max(rect1.left, rect2.left))
- Y_overlap = max(0, min(rect1.bottom, rect2.bottom) - max(rect1.top, rect2.top))
- OverlapArea = x_overlap y_overlap
These formulas calculate the overlap in the x and y directions and then multiply them together to get the total overlap area.
It's important to note that this solution only works for non-rotated rectangles. If the rectangles are allowed to rotate, the problem becomes more complex, and different methods, such as trigonometric calculations, may be required to determine the intersection area.
Additionally, when dealing with multiple cameras, you can use feature points and homography matrices to align the images and create panoramic shots.
The Lasting Legacy of the Pd16 Plenax Folding Camera
You may want to see also
Draw rectangles in different colours and calculate purple pixels
To draw rectangles in different colours and calculate purple pixels, you can use the Python library Pygame, which allows you to draw various shapes like rectangles, circles, polygons, and lines. Here's an example code snippet to get you started:
Python
Import pygame
Pygame.init()
Screen = pygame.display.set_mode((500, 500))
Drawing a red rectangle
Color = (255, 0, 0) # Red colour
Rect = (10, 10, 100, 50) # Rectangle position and size
Pygame.draw.rect(screen, color, rect)
Drawing a green rectangle
Color = (0, 255, 0) # Green colour
Rect = (50, 50, 100, 50) # Rectangle position and size
Pygame.draw.rect(screen, color, rect)
Drawing a blue rectangle
Color = (0, 0, 255) # Blue colour
Rect = (100, 100, 100, 50) # Rectangle position and size
Pygame.draw.rect(screen, color, rect)
Pygame.display.flip()
In this code, we first import the `pygame` library and initialise a screen with a size of 500x500 pixels. We then define the colour and position of each rectangle before using the `pygame.draw.rect()` function to draw them on the screen. Finally, `pygame.display.flip()` updates the screen to display the drawn shapes.
To calculate the number of purple pixels, you can use image processing techniques. First, you need to determine the RGB values for purple. In the RGB colour model, purple is obtained by mixing equal amounts of red and blue while excluding green. So, for a light shade of purple, the RGB values would be (255, 0, 255), and for a darker shade, it would be (102, 0, 102). Once you have defined the RGB values for your desired shade of purple, you can iterate through each pixel in the image and compare its colour to the RGB values of purple. For each pixel that matches the purple colour, increment a counter. This counter will give you the total number of purple pixels in the image.
Focusing Your Camera on FaceTime: Tips and Tricks
You may want to see also
Frequently asked questions
The Sutherland-Hodgman polygon clipping algorithm can be used to find the overlapping area of two rotated rectangles. This algorithm clips one of the polygons with the four supporting lines (half-planes) of the other polygon. The intersection area can then be found by calculating the area of the resulting polygon using the polygon area formula.
The overlapping ratio of two rotated rectangles can be calculated by first finding the intersection area of the two rectangles and then dividing it by the area of one of the rectangles. The intersection area can be found using the Sutherland-Hodgman polygon clipping algorithm.
To compute the overlapping area of two rotated squares, you can find the unshaded area and subtract it from the area of the square. This can be done by using trigonometric functions and the given angle of rotation.
Yes, an alternative method is to draw and overlay the two rectangles in different colours (e.g. red and blue) and then calculate the number of pixels that are a combination of both colours (e.g. purple). This method may be less accurate and more time-consuming, especially for large images.