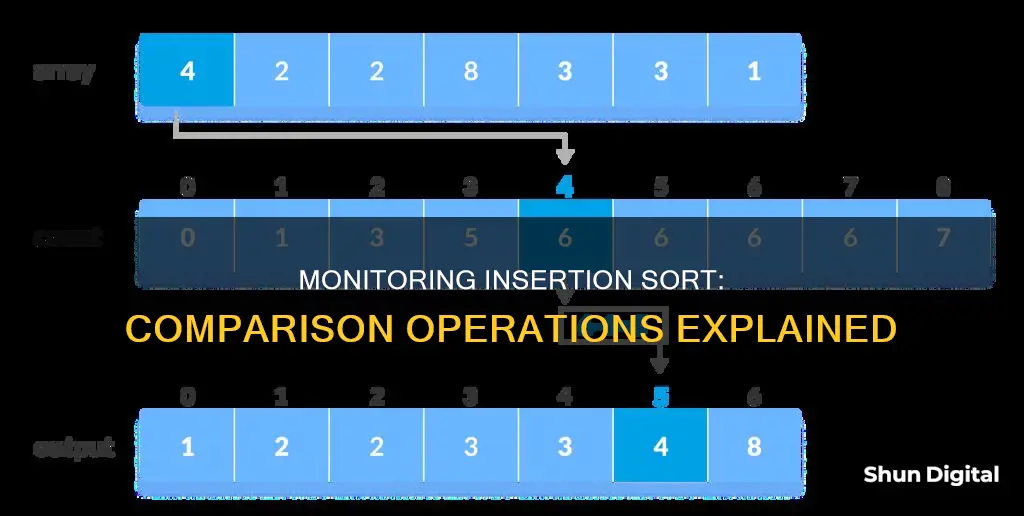
Insertion sort is a simple algorithm that sorts a list by iteratively building a sorted list, one item at a time, through comparisons. The algorithm works by removing the first item from an unsorted list and marking it as the item to be inserted. It then works backward from the end of the sorted list, comparing the item to be inserted with the current item. This process is repeated until the unsorted list is empty, resulting in a fully sorted list.
The number of comparisons required by insertion sort depends on the original order of the items. The best-case scenario is when the list is already sorted, requiring only one comparison per pass. On the other hand, the worst-case scenario is when the list is in reverse sorted order, necessitating the maximum number of comparisons.
To monitor the comparisons performed in insertion sort, a counter can be implemented within the algorithm. This counter would increment each time a comparison is made between the item to be inserted and the current item in the sorted list. By tracking this count, one can gain insights into the efficiency of the insertion sort for a given input list.
Characteristics | Values |
---|---|
Time complexity | O(n^2) |
Best case | O(n) |
Worst case | O(n^2) |
Average case | O(n^2) |
Number of comparisons | Depends on the original order of the items |
Minimum number of comparisons | n-1 |
Maximum number of comparisons | 1/2(n^2-n) |
Average number of comparisons | 1/4(n^2-n) |
What You'll Learn
Counting comparisons in insertion sort
Insertion sort is a simple sorting algorithm that builds a final sorted array by making comparisons. The number of comparisons required to sort N items using the insertion method depends on the original order of the items.
Insertion sort removes the first item from the unsorted list and marks it as the item to be inserted. It then works backward from the sorted list, comparing the item to be inserted with the current item. The algorithm continues moving backward until it reaches the beginning of the list or encounters an item that is less than or equal to the item to be inserted. At this point, the item is inserted at the current insertion point. This process is repeated until the unsorted list is empty.
The number of comparisons in insertion sort can vary depending on the initial order of the list. When the input sequence is in reverse order, it is the worst case for insertion sort as each new item added to the sorted partition has to be compared to every other item in the partition. In this case, the number of comparisons is given by the formula:
> $\frac{n(n - 1)}{2}$
For example, sorting 10 items in reverse order would require 45 comparisons.
On the other hand, when the input sequence is already sorted, insertion sort requires far fewer comparisons. In this case, the last item in the sorted list will always be less than or equal to the first item in the unsorted list, so only one comparison is needed during each pass to find the insertion point. Thus, the total number of comparisons necessary for insertion sort in this best-case scenario is N - 1.
The average case for insertion sort also depends on the initial order of the list. Sometimes the insertion point will appear at or near the end of the list, requiring only a few comparisons. Other times, the insertion point will be near the beginning of the sorted list, necessitating a comparison between the item to be inserted and most or all of the items in the sorted list. On average, these good and bad cases tend to "cancel" each other out, resulting in an average number of comparisons per pass of 1/4 N.
In summary, the minimum, maximum, and average number of insertion sort comparisons for N items can be expressed as follows:
- Minimum: N - 1
- Maximum: 1/2(N^2 - N)
- Average: 1/4(N^2 - N)
When comparing insertion sort to other sorting algorithms, the average case formula is typically used to represent its expected performance.
Insertion sort is much less efficient for large lists compared to more advanced algorithms like quicksort, heapsort, or merge sort. However, it provides several advantages, including simplicity of implementation, efficiency for small data sets, and adaptability to data sets that are already substantially sorted.
Asus Monitors: VESA Mount Compatibility and Your Options
You may want to see also
Comparison count in insertion sort implementation
The insertion sort algorithm is a simple sorting algorithm that builds a final sorted array or list through a series of comparisons. It is often used by people when manually sorting a hand of cards.
The algorithm works by dividing an input list into two parts: a "sorted list" consisting of one item (the first item in the input list), and an "unsorted list" consisting of all the remaining items. It then removes the first item from the unsorted list and works its way backward through the sorted list, comparing the removed item to each item in the sorted list. If the item from the unsorted list is smaller than the item in the sorted list, it is inserted into the current position in the sorted list, and the process is repeated until the unsorted list is empty.
The number of comparisons required by insertion sort depends on the original order of the items. If the input list is already sorted, only one comparison is needed on each pass, resulting in a linear running time (O(n)). On the other hand, if the input list is in reverse sorted order (the worst-case scenario), insertion sort requires the maximum number of comparisons, resulting in a quadratic running time (O(n^2)).
In the average case, insertion sort requires approximately half as many comparisons as selection sort. This is because insertion sort only requires a single comparison when the (k+1)-st element is greater than the k-th element, whereas selection sort must always scan all remaining elements to find the smallest element.
When implementing insertion sort, it is important to keep track of the number of comparisons made during the sorting process. This can be done by incrementing a "comparisons" variable inside the loop that performs the comparisons. However, it is important to only increment the variable when a successful comparison is made (i.e., when a swap is needed) to ensure an accurate count.
Additionally, when counting comparisons, it is important to note that only comparisons between data values in the input list are considered. Comparisons involving indices, such as checking if "j > 0", are not typically counted as comparisons in the context of evaluating sorting algorithms.
Curved Monitors: Why the Hype?
You may want to see also
Best and worst-case comparison counts
The number of comparisons required to sort N items using the insertion method depends on the original order of the items.
The best-case scenario for insertion sort is when the input array is already sorted. In this case, insertion sort has a linear running time (i.e., O(n)). During each iteration, the first remaining element of the input is only compared with the rightmost element of the sorted subsection of the array. This results in a minimum number of insertion sort comparisons, which can be calculated using the formula: Minimum number of insertion sort comparisons = N - 1.
On the other hand, the worst-case scenario for insertion sort occurs when the input array is in reverse sorted order. In this case, the insertion point will always be at the beginning of the sorted list, forcing the algorithm to examine and compare every item in the sorted list to the item to be inserted during each pass. The number of comparisons will be N - 1 times the average length of the sorted list, which is approximately half of N. This results in a maximum number of insertion sort comparisons, calculated using the formula: Maximum number of insertion sort comparisons = 1/2(N^2 - N).
The average case for insertion sort also requires a quadratic number of comparisons, making it impractical for sorting large arrays. However, insertion sort is one of the fastest algorithms for sorting very small arrays, even faster than quicksort. The average number of comparisons per pass is one-fourth of N, and the total number of insertion sort comparisons in the average case can be calculated using the formula: Average number of insertion sort comparisons = 1/4(N^2 - N).
To summarise, the number of comparisons required by insertion sort varies depending on the original order of the items. The best case occurs when the list is already sorted, the worst case occurs when the list is in reverse sorted order, and the average case falls somewhere in between.
Monitoring Tomcat Memory Usage: A Comprehensive Guide
You may want to see also
Comparison count in different sorting algorithms
The number of comparisons required by a sorting algorithm is an important factor in determining its efficiency. The comparison count varies depending on the algorithm used and the original order of the items to be sorted.
Insertion sort is a simple algorithm that builds the final sorted array by comparing and inserting elements one at a time. It is efficient for small datasets and when the data is already substantially sorted. The number of comparisons in insertion sort depends on the initial order of the elements. For example, if the items are already sorted, only one comparison is needed to confirm the order, resulting in a linear running time of O(n). However, in the worst-case scenario, where the items are sorted in reverse order, insertion sort requires a quadratic running time of O(n^2).
Other popular sorting algorithms include selection sort, bubble sort, merge sort, and quicksort. Each of these algorithms has its own comparison count characteristics.
Selection sort, like insertion sort, has a worst-case time complexity of O(n^2). It achieves this by finding the minimum value and repeatedly swapping it with the value in the first position until the entire list is sorted.
Bubble sort is another simple algorithm but is generally inefficient due to its O(n^2) time complexity. It works by comparing adjacent elements and swapping them if they are in the wrong order. This process is repeated for each pair of elements until the list is sorted.
Merge sort and quicksort, on the other hand, have better average-case and worst-case time complexities of O(n log n). Merge sort achieves this by dividing the list into smaller sublists, sorting them, and then merging them back together. Quicksort is a divide-and-conquer algorithm that selects a pivot element and partitions the list into two sublists, recursively sorting each sublist.
The efficiency of a sorting algorithm is not solely determined by the number of comparisons but also by other factors such as swaps, memory usage, stability, and adaptability. However, understanding the comparison count of different sorting algorithms provides valuable insights into their performance characteristics.
Beating a Scram Ankle Monitor: Strategies and Potential Consequences
You may want to see also
Comparison count in different input sequences
The number of comparisons required by insertion sort depends on the original order of the items. The best-case scenario for insertion sort is when the input sequence is already sorted. In this case, the algorithm only needs to make one comparison per pass, resulting in a linear running time of O(n). For example, if you have a list of 10 items that are already sorted, insertion sort will only require 9 comparisons to complete the sorting process.
On the other hand, the worst-case scenario for insertion sort occurs when the input sequence is in reverse order. In this case, each new item added to the sorted partition needs to be compared to every other item in the partition, resulting in a quadratic running time of O(n^2). For instance, with 10 items in reverse order, insertion sort would require 45 comparisons. Each item, except the first one, has to be compared to all the items before it in the sorted partition.
The average case for insertion sort falls somewhere between the best and worst-case scenarios. The number of comparisons in the average case is given by the formula: O(n^2)/4. This means that for a list of 10 items, the average number of comparisons would be around 22.5.
It's important to note that the number of comparisons in insertion sort can vary depending on the specific input sequence. The more sorted the input sequence is, the fewer comparisons are needed. Conversely, if the input sequence is in reverse order or nearly reverse order, the number of comparisons increases significantly.
Monitoring Kids' Social Media: Parenting in the Digital Age
You may want to see also
Frequently asked questions
The number of comparisons in insertion sort depends on the initial order of the list. The best-case scenario is when the list is already sorted, requiring only one comparison per pass, resulting in n-1 comparisons. The worst-case scenario is when the list is in reverse sorted order, necessitating n-1 passes and comparing the item to be inserted with every item in the sorted list. This results in a maximum of (n-1) x (n/2) comparisons.
Insertion sort is a simple algorithm that builds a sorted array or list one item at a time through comparisons. It starts by assuming a list with one item is already sorted. On each pass, the current item is checked against those in the sorted sublist, and items that are greater are shifted to the right. This process continues until the item is inserted in its correct position.
Insertion sort is efficient for small datasets and lists that are already substantially sorted. It is more efficient in practice than other simple quadratic algorithms like selection or bubble sort. However, it is much less efficient on large lists compared to more advanced algorithms such as quicksort, heapsort, or merge sort.
The time complexity of insertion sort is O(n^2) in the average and worst cases, making it impractical for sorting large arrays. However, it has a linear running time of O(n) in the best-case scenario when the list is already sorted.